使用java设计一个关于继承关系的类结构,并在此结构中实现方法多态(重载/重写)和类型多态
时间: 2024-10-22 13:19:26 浏览: 28
在Java中,我们可以创建一个简单的继承结构来展示方法多态(重载和重写)以及类型多态(泛型)。首先,我们定义一个父类`Shape`:
```java
public abstract class Shape {
protected String color; // 共享属性
public Shape(String color) {
this.color = color;
}
// 方法重载示例 - 形状的面积计算
public abstract double getArea(); // 纯虚函数
// 属性打印方法
public void printInfo() {
System.out.println("Color: " + color);
}
}
```
接着,我们创建两个子类,分别继承自`Shape`并实现`getArea()`方法,一个是矩形`Rectangle`,另一个是圆形`Circle`:
```java
public class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(String color, double width, double height) {
super(color);
this.width = width;
this.height = height;
}
@Override
public double getArea() {
return width * height;
}
}
public class Circle extends Shape {
private double radius;
public Circle(String color, double radius) {
super(color);
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * Math.pow(radius, 2);
}
}
```
在这里,`getArea()`方法就是方法重写,每个子类都有自己的实现。同时,由于它们都是`Shape`类型的引用,你可以根据需要实例化任何一个子类的对象并调用`getArea()`,这就是类型多态。
示例使用:
```java
public class Main {
public static void main(String[] args) {
Shape rectangle = new Rectangle("Red", 5, 4);
Shape circle = new Circle("Blue", 3);
System.out.println(rectangle.getArea()); // 输出矩形的面积
System.out.println(circle.getArea()); // 输出圆的面积
rectangle.printInfo();
circle.printInfo();
}
}
```
阅读全文
相关推荐
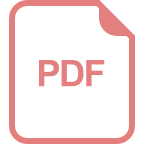
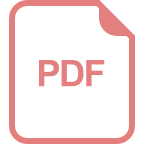

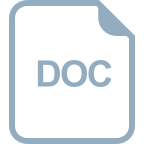
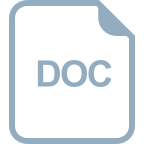
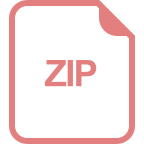
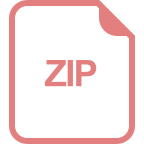
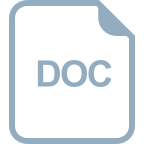
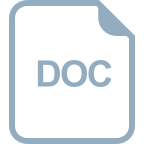
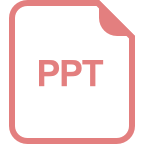
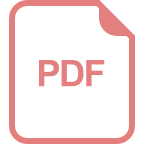
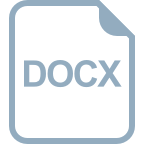
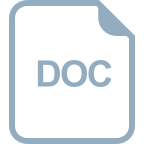
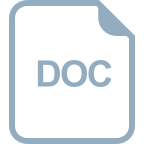
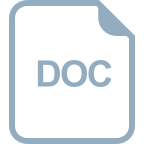
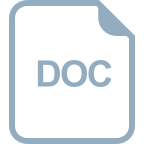
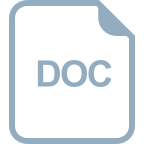
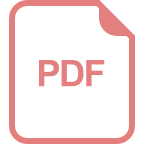