用python编一个飞机的小游戏
时间: 2024-09-22 10:01:59 浏览: 37
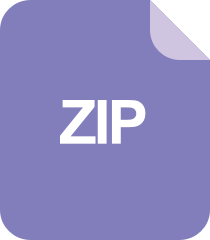
用Python写一个飞机大战小游戏

要用Python编写一个飞机小游戏,你可以使用pygame库。首先,你需要安装pygame库,可以通过以下命令安装:
```bash
pip install pygame
```
接下来,我将为你提供一个简单的飞机游戏示例代码:
```python
import pygame
import sys
# 初始化pygame
pygame.init()
# 设置屏幕大小
screen_width = 480
screen_height = 640
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏标题
pygame.display.set_caption("飞机小游戏")
# 加载飞机图片
plane_image = pygame.image.load("plane.png")
plane_rect = plane_image.get_rect()
plane_rect.x = (screen_width - plane_rect.width) // 2
plane_rect.y = screen_height - plane_rect.height - 50
# 游戏主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 获取按键状态
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
plane_rect.x -= 5
if keys[pygame.K_RIGHT]:
plane_rect.x += 5
if keys[pygame.K_UP]:
plane_rect.y -= 5
if keys[pygame.K_DOWN]:
plane_rect.y += 5
# 限制飞机在屏幕范围内
if plane_rect.x < 0:
plane_rect.x = 0
if plane_rect.x > screen_width - plane_rect.width:
plane_rect.x = screen_width - plane_rect.width
if plane_rect.y < 0:
plane_rect.y = 0
if plane_rect.y > screen_height - plane_rect.height:
plane_rect.y = screen_height - plane_rect.height
# 绘制背景和飞机
screen.fill((255, 255, 255))
screen.blit(plane_image, plane_rect)
# 更新屏幕显示
pygame.display.flip()
```
注意:你需要将"plane.png"替换为你自己的飞机图片文件路径。此外,你还可以添加更多功能,如敌人、子弹、碰撞检测等,以使游戏更加有趣。
阅读全文
相关推荐
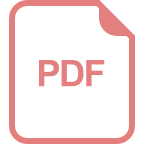
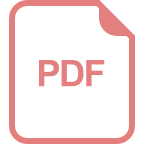






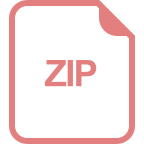
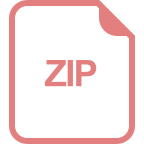
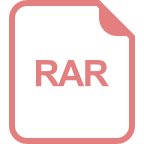
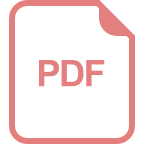
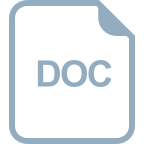
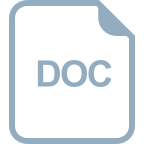
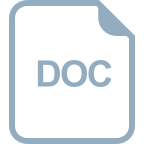
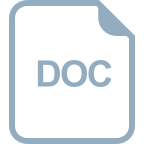

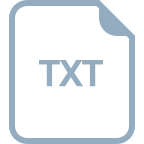