给我用python编一个f飞机大战游戏
时间: 2024-01-07 11:04:11 浏览: 78
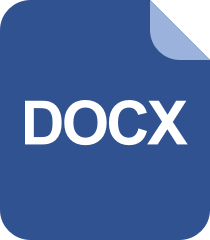
Python编写飞机大战游戏
好的,下面是一个简单的飞机大战游戏的Python代码:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 设置游戏窗口大小
screen_width = 480
screen_height = 700
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏标题
pygame.display.set_caption("飞机大战")
# 加载背景图片
bg = pygame.image.load("images/background.png")
# 加载飞机图片
player_img = pygame.image.load("images/me1.png")
# 加载子弹图片
bullet_img = pygame.image.load("images/bullet1.png")
# 加载敌机图片
enemy_img = pygame.image.load("images/enemy1.png")
# 设置字体
font = pygame.font.SysFont(None, 48)
# 设置游戏结束状态
game_over = False
# 定义函数,用于绘制文本
def draw_text(text, color, x, y):
text_surface = font.render(text, True, color)
text_rect = text_surface.get_rect()
text_rect.center = (x, y)
screen.blit(text_surface, text_rect)
# 定义函数,用于绘制玩家飞机
def draw_player(x, y):
screen.blit(player_img, (x, y))
# 定义函数,用于绘制敌机
def draw_enemy(x, y):
screen.blit(enemy_img, (x, y))
# 定义函数,用于绘制子弹
def draw_bullet(x, y):
screen.blit(bullet_img, (x, y))
# 定义函数,用于检测子弹与敌机是否相撞
def collision(bullet_x, bullet_y, enemy_x, enemy_y):
distance = ((bullet_x - enemy_x) ** 2 + (bullet_y - enemy_y) ** 2) ** 0.5
if distance < 27:
return True
else:
return False
# 定义函数,用于播放游戏音效
def play_sound(sound):
sound.play()
# 加载游戏音效
bullet_sound = pygame.mixer.Sound("sounds/bullet.wav")
enemy_sound = pygame.mixer.Sound("sounds/enemy.wav")
gameover_sound = pygame.mixer.Sound("sounds/gameover.wav")
# 设置玩家飞机的初始位置
player_x = 200
player_y = 500
# 设置敌机的初始位置
enemy_x = random.randint(0, screen_width - 50)
enemy_y = 0
# 子弹的初始位置和状态
bullet_x = 0
bullet_y = 0
bullet_state = "ready"
# 分数和生命值
score = 0
lives = 3
# 游戏循环
while not game_over:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
player_x -= 5
elif event.key == pygame.K_RIGHT:
player_x += 5
elif event.key == pygame.K_SPACE:
if bullet_state == "ready":
bullet_x = player_x + 16
bullet_y = player_y - 30
bullet_state = "fire"
play_sound(bullet_sound)
# 绘制背景图片
screen.blit(bg, (0, 0))
# 绘制玩家飞机
draw_player(player_x, player_y)
# 绘制敌机
draw_enemy(enemy_x, enemy_y)
# 移动敌机
enemy_y += 5
# 如果敌机飞出屏幕,则重新生成敌机
if enemy_y > screen_height:
enemy_x = random.randint(0, screen_width - 50)
enemy_y = 0
lives -= 1
play_sound(enemy_sound)
# 绘制分数和生命值
draw_text("Score: " + str(score), (255, 255, 255), 100, 30)
draw_text("Lives: " + str(lives), (255, 255, 255), 380, 30)
# 如果子弹状态为 "fire",则移动子弹并检测是否与敌机相撞
if bullet_state == "fire":
draw_bullet(bullet_x, bullet_y)
bullet_y -= 10
if bullet_y < 0:
bullet_state = "ready"
elif collision(bullet_x, bullet_y, enemy_x, enemy_y):
bullet_state = "ready"
score += 10
enemy_x = random.randint(0, screen_width - 50)
enemy_y = 0
play_sound(enemy_sound)
# 检测玩家飞机与敌机是否相撞
distance = ((player_x - enemy_x) ** 2 + (player_y - enemy_y) ** 2) ** 0.5
if distance < 60:
lives -= 1
enemy_x = random.randint(0, screen_width - 50)
enemy_y = 0
play_sound(enemy_sound)
# 如果生命值为 0,则游戏结束
if lives == 0:
game_over = True
play_sound(gameover_sound)
draw_text("Game Over", (255, 0, 0), screen_width // 2, screen_height // 2)
# 更新屏幕显示
pygame.display.update()
# 退出 Pygame
pygame.quit()
```
使用方法:
1.将代码保存为“plane.py”文件
2.在代码所在目录下创建一个名为“images”的文件夹,将游戏所需的图片文件放在这个文件夹中
3.在代码所在目录下创建一个名为“sounds”的文件夹,将游戏所需的音效文件放在这个文件夹中
4.运行“plane.py”文件,开始游戏
阅读全文
相关推荐
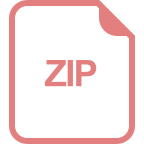
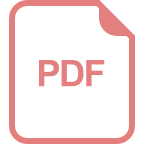


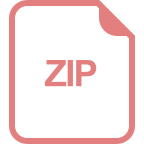
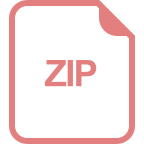
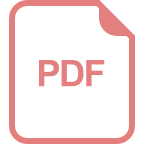
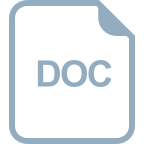



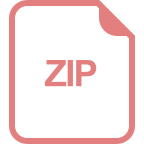
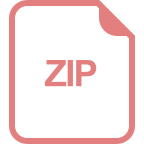
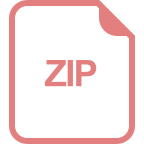
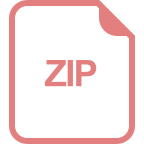
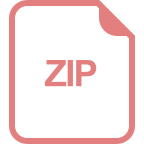
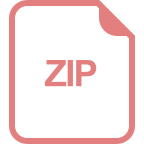
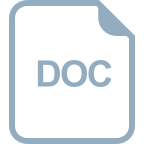