以上服务端代码和客户端代码请补充完整并写好注释
时间: 2024-10-25 19:13:59 浏览: 9
为了帮助您理解,我需要了解您希望完成的具体服务端和客户端代码是什么类型的项目,比如web应用、移动应用还是游戏服务器等。通常,我会给出一个简单的示例,比如HTTP RESTful API服务端和JavaScript客户端调用。
**服务端(假设是Node.js + Express框架)**:
```javascript
// 引入必要的模块
const express = require('express');
const bodyParser = require('body-parser');
// 创建Express应用
const app = express();
// 使用body-parser中间件解析请求体
app.use(bodyParser.json());
// 定义API路由,这里我们创建一个用于添加用户的接口
// POST /users - 添加新用户
app.post('/users', (req, res) => {
const newUser = req.body; // 获取请求体中的用户数据
try {
// 这里只是一个模拟数据库操作,实际会连接到数据库
// 新增用户
addUserToDatabase(newUser);
res.status(201).json({ message: 'User added successfully.' });
} catch (error) {
res.status(500).json({ error: 'Failed to add user.' });
}
});
// 模拟函数,实际操作应该替换为数据库操作
function addUserToDatabase(user) {
console.log(`Adding user with data: ${JSON.stringify(user)}`);
}
// 启动服务器
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
```
**客户端(假设是HTML+JavaScript)**:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
</head>
<body>
<button id="addUserButton">Add User</button>
<!-- 异步处理按钮点击事件 -->
<script>
$(document).ready(() => {
$('#addUserButton').click(() => {
const newUserData = { name: 'John Doe', email: 'john@example.com' };
$.ajax({
url: '/users',
type: 'POST',
contentType: 'application/json',
data: JSON.stringify(newUserData),
success: function(response) {
console.log('Success:', response.message);
},
error: function(error) {
console.error('Error:', error.responseJSON.error);
}
});
});
});
</script>
</body>
</html>
```
这个例子是一个基本的RESTful API服务端和一个发送POST请求的简单客户端。
阅读全文
相关推荐
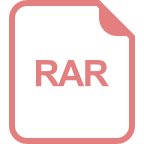
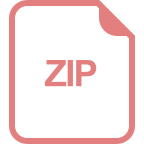
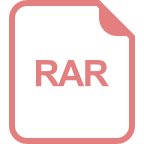
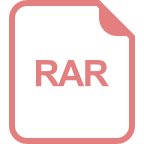
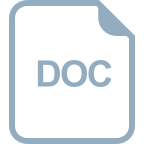
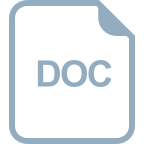
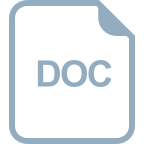
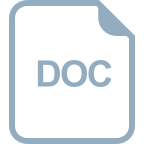
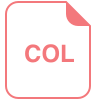
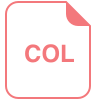
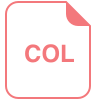
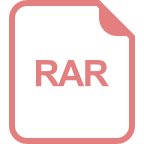
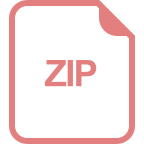
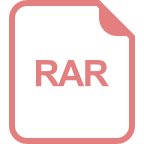
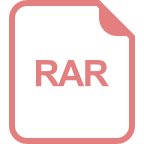
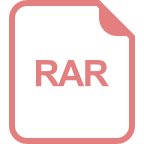