c++ format_string
时间: 2023-10-07 15:04:51 浏览: 65
在C++中,可以使用stringstream类来格式化字符串。你可以将不同类型的数据插入到stringstream对象中,并使用<<操作符来拼接它们。最后,通过调用sstream对象的str()方法,将其转换为字符串。
在C中,你可以使用FormatString函数来格式化字符串。这个函数的原理是使用变量参数列表和_vsnprintf_s函数来将格式化后的字符串存储到一个字符数组中。然后,将这个字符数组转换为string类型的字符串并返回。
此外,在C中也有一个名为FormatWstring的函数,它的原理与FormatString相似,但用于处理宽字符串。
因此,无论你是在C++中还是在C中,都可以使用相应的方法来格式化字符串。
相关问题
std::format_to_string
std::format_to_string是C++20中引入的一个函数模板,用于将格式化的数据转换为字符串。它位于<format>头文件中,并且属于std命名空间。
std::format_to_string函数的语法如下:
```cpp
template <typename... Args>
std::string format_to_string(const std::string& format_str, const Args&... args);
```
该函数接受一个格式化字符串format_str和一系列参数args,并返回一个包含格式化结果的std::string对象。
使用std::format_to_string函数,你可以通过在格式化字符串中使用占位符{}来指定参数的位置,并在args中传递相应的值。例如:
```cpp
#include <format>
#include <iostream>
int main() {
std::string name = "Alice";
int age = 25;
std::string result = std::format_to_string("My name is {} and I am {} years old.", name, age);
std::cout << result << std::endl;
return 0;
}
```
输出结果为:
```
My name is Alice and I am 25 years old.
```
在上面的例子中,我们使用了两个占位符{}来表示两个参数的位置,分别是name和age。通过传递相应的值,我们得到了格式化后的字符串。
需要注意的是,std::format_to_string函数在C++20中才被引入,因此在旧版本的编译器中可能无法使用。如果你使用的是旧版本的编译器,可以考虑使用第三方库,如fmt库来实现类似的功能。
/usr/include/spdlog/logger.h:90:9: required from ‘void spdlog::logger::log(spdlog::source_loc, spdlog::level::level_enum, fmt::v8::format_string<T ...>, Args&& ...) [with Args = {cv::Rect_<int>&}; fmt::v8::format_string<T ...> = fmt::v8::basic_format_string<char, cv::Rect_<int>&>]’
这个错误提示出现在 `spdlog` 库的 `logger.h` 文件中,它表示在使用 `spdlog` 记录日志时,传递了一个 `cv::Rect_<int>&` 类型的参数,这个类型无法被格式化输出。具体来说,`fmt` 库不知道如何将这个类型转换成字符串,所以编译器报错了。
为了解决这个问题,你需要为 `cv::Rect_<int>&` 类型提供一个格式化器,告诉 `fmt` 库如何将它转换成字符串。具体的做法是,在你的代码中实现一个 `formatter<cv::Rect_<int>&>` 类型的类,然后在这个类中实现 `format` 函数,将 `cv::Rect_<int>&` 类型转换成字符串。然后将这个格式化器传递给 `spdlog::logger` 对象,让它在记录日志时使用这个格式化器。
下面是一个示例:
```c++
#include <fmt/format.h>
#include <spdlog/spdlog.h>
template <>
struct fmt::formatter<cv::Rect_<int>&> {
template <typename ParseContext>
constexpr auto parse(ParseContext& ctx) { return ctx.begin(); }
template <typename FormatContext>
auto format(const cv::Rect_<int>& rect, FormatContext& ctx) {
return fmt::format_to(ctx.out(), "{{ x:{}, y:{}, width:{}, height:{} }}", rect.x, rect.y, rect.width, rect.height);
}
};
int main() {
auto logger = spdlog::stdout_logger_mt("console");
cv::Rect_<int> rect(10, 20, 30, 40);
logger->info("Rect: {}", rect);
return 0;
}
```
在这个示例中,我们为 `cv::Rect_<int>&` 类型提供了一个格式化器 `formatter<cv::Rect_<int>&>`,然后在使用 `spdlog` 记录日志时,传递了一个 `cv::Rect_<int>&` 类型的参数,让它使用我们自己实现的格式化器输出日志。
阅读全文
相关推荐
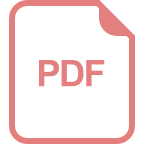
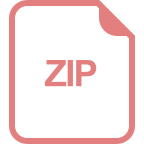
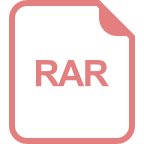













