员工考勤管理系统java
时间: 2023-08-22 19:10:24 浏览: 192
员工考勤管理系统可以使用Java进行开发。Java 是一种广泛使用的编程语言,具有强大的面向对象特性和丰富的开发工具和框架,非常适合用于构建企业级应用程序。
在Java中,你可以使用Java的核心类库和其他第三方库来处理员工考勤管理系统的各个方面,例如员工信息管理、考勤记录、统计分析等功能。你可以使用Java的数据库访问技术(如JDBC)来连接数据库并存储员工的信息和考勤记录。同时,你可以使用Java的图形用户界面(GUI)库(如Swing或JavaFX)来创建用户友好的界面,方便用户进行操作和查看考勤信息。
此外,Java还有众多开发框架和工具可以帮助简化开发过程,例如Spring框架、Hibernate ORM框架等,它们可以提供更高级的功能和便利性。
总结来说,使用Java开发员工考勤管理系统可以利用Java的强大特性和丰富的生态系统来实现各种功能,并提供稳定可靠的应用程序。
相关问题
校职工考勤管理系统java源码
校职工考勤管理系统的Java源码,是用Java编程语言编写的管理系统程序代码。这个系统主要功能是统计、记录和管理校内职工的考勤信息,包括上下班打卡记录、工作时间统计、请假流程审批等等。这种管理系统结合了计算机技术和管理科学的优势,旨在提高校内职工的工作效率,以及减少人工管理的成本。
在Java源码中,包含了各个功能模块的实现代码、相关数据结构和算法代码。比如说,工作时间统计模块可以用时间戳进行计算,记录职工的上班时间和下班时间,并将时间差输出为工作时长。请假流程审批模块可以用有限状态机或图论算法进行设计,对于不同类型的请假申请,系统自动根据规则判断并分配给相应的审批人。
此外,这个管理系统还涉及到数据库技术,用于存储职工的考勤信息和个人档案资料。在Java源码中,包含了与数据库交互的代码和SQL语句,用于实现数据的增删改查操作。
总之,校职工考勤管理系统的Java源码,是一个涉及多个技术领域的程序代码,在实现职工考勤管理的同时,也为我们提供了一个学习和理解各种技术的良好机会。
公司员工考勤管理系统java代码实现
公司员工考勤管理系统的Java实现包括以下步骤:
1. 定义员工类和考勤类,员工类包含员工的基本信息,考勤类包含员工的考勤记录。
```java
public class Employee {
private int id;
private String name;
private String department;
private String position;
// 省略getter和setter方法
}
public class Attendance {
private int id;
private Date date;
private int employeeId;
private String status;
// 省略getter和setter方法
}
```
2. 定义数据库连接类,实现与数据库的连接和操作。
```java
public class DBConnection {
private static final String URL = "jdbc:mysql://localhost:3306/attendance";
private static final String USER = "root";
private static final String PASSWORD = "root";
public static Connection getConnection() {
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(URL, USER, PASSWORD);
} catch (Exception e) {
e.printStackTrace();
}
return conn;
}
public static void close(Connection conn, Statement stmt, ResultSet rs) {
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
3. 定义员工管理类和考勤管理类,实现员工和考勤的增删改查等操作。
```java
public class EmployeeManager {
public void addEmployee(Employee employee) {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DBConnection.getConnection();
String sql = "INSERT INTO employee (id, name, department, position) VALUES (?, ?, ?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, employee.getId());
stmt.setString(2, employee.getName());
stmt.setString(3, employee.getDepartment());
stmt.setString(4, employee.getPosition());
stmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
DBConnection.close(conn, stmt, null);
}
}
public void deleteEmployee(int id) {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DBConnection.getConnection();
String sql = "DELETE FROM employee WHERE id=?";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, id);
stmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
DBConnection.close(conn, stmt, null);
}
}
public void updateEmployee(Employee employee) {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DBConnection.getConnection();
String sql = "UPDATE employee SET name=?, department=?, position=? WHERE id=?";
stmt = conn.prepareStatement(sql);
stmt.setString(1, employee.getName());
stmt.setString(2, employee.getDepartment());
stmt.setString(3, employee.getPosition());
stmt.setInt(4, employee.getId());
stmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
DBConnection.close(conn, stmt, null);
}
}
public List<Employee> getEmployees() {
List<Employee> employees = new ArrayList<>();
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
conn = DBConnection.getConnection();
String sql = "SELECT * FROM employee";
stmt = conn.prepareStatement(sql);
rs = stmt.executeQuery();
while (rs.next()) {
Employee employee = new Employee();
employee.setId(rs.getInt("id"));
employee.setName(rs.getString("name"));
employee.setDepartment(rs.getString("department"));
employee.setPosition(rs.getString("position"));
employees.add(employee);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
DBConnection.close(conn, stmt, rs);
}
return employees;
}
}
public class AttendanceManager {
public void addAttendance(Attendance attendance) {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DBConnection.getConnection();
String sql = "INSERT INTO attendance (id, date, employee_id, status) VALUES (?, ?, ?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, attendance.getId());
stmt.setDate(2, new java.sql.Date(attendance.getDate().getTime()));
stmt.setInt(3, attendance.getEmployeeId());
stmt.setString(4, attendance.getStatus());
stmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
DBConnection.close(conn, stmt, null);
}
}
public void deleteAttendance(int id) {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DBConnection.getConnection();
String sql = "DELETE FROM attendance WHERE id=?";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, id);
stmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
DBConnection.close(conn, stmt, null);
}
}
public void updateAttendance(Attendance attendance) {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DBConnection.getConnection();
String sql = "UPDATE attendance SET date=?, employee_id=?, status=? WHERE id=?";
stmt = conn.prepareStatement(sql);
stmt.setDate(1, new java.sql.Date(attendance.getDate().getTime()));
stmt.setInt(2, attendance.getEmployeeId());
stmt.setString(3, attendance.getStatus());
stmt.setInt(4, attendance.getId());
stmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
DBConnection.close(conn, stmt, null);
}
}
public List<Attendance> getAttendances() {
List<Attendance> attendances = new ArrayList<>();
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
conn = DBConnection.getConnection();
String sql = "SELECT * FROM attendance";
stmt = conn.prepareStatement(sql);
rs = stmt.executeQuery();
while (rs.next()) {
Attendance attendance = new Attendance();
attendance.setId(rs.getInt("id"));
attendance.setDate(rs.getDate("date"));
attendance.setEmployeeId(rs.getInt("employee_id"));
attendance.setStatus(rs.getString("status"));
attendances.add(attendance);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
DBConnection.close(conn, stmt, rs);
}
return attendances;
}
}
```
4. 编写测试类,测试员工和考勤的增删改查等操作。
```java
public class Test {
public static void main(String[] args) {
EmployeeManager employeeManager = new EmployeeManager();
AttendanceManager attendanceManager = new AttendanceManager();
// 添加员工
Employee employee1 = new Employee(1, "张三", "研发部", "工程师");
employeeManager.addEmployee(employee1);
Employee employee2 = new Employee(2, "李四", "市场部", "销售");
employeeManager.addEmployee(employee2);
// 添加考勤记录
Attendance attendance1 = new Attendance(1, new Date(), 1, "正常");
attendanceManager.addAttendance(attendance1);
Attendance attendance2 = new Attendance(2, new Date(), 2, "迟到");
attendanceManager.addAttendance(attendance2);
// 查询员工列表
List<Employee> employees = employeeManager.getEmployees();
for (Employee employee : employees) {
System.out.println(employee.getId() + " " + employee.getName() + " " + employee.getDepartment() + " " + employee.getPosition());
}
// 查询考勤记录列表
List<Attendance> attendances = attendanceManager.getAttendances();
for (Attendance attendance : attendances) {
System.out.println(attendance.getId() + " " + attendance.getDate() + " " + attendance.getEmployeeId() + " " + attendance.getStatus());
}
// 更新员工信息
Employee employee3 = new Employee(2, "王五", "市场部", "销售经理");
employeeManager.updateEmployee(employee3);
// 删除考勤记录
attendanceManager.deleteAttendance(2);
// 删除员工
employeeManager.deleteEmployee(1);
}
}
```
以上是一个简单的公司员工考勤管理系统的Java实现方案,其中还有很多细节需要处理,例如数据库连接池、异常处理、日期格式化等。
阅读全文
相关推荐
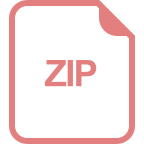
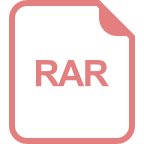
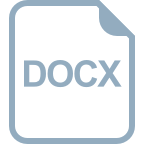













