如何使用Python编写代码从链家网站上抓取租房相关的房产数据?
时间: 2024-10-17 08:08:41 浏览: 74
要从链家网站抓取租房相关的房产数据,你需要使用Python的一些网络爬虫库,如`requests`, `BeautifulSoup`或`Scrapy`。这里我将提供一个基础的示例,说明如何使用`requests`和`BeautifulSoup`来获取网页源码,然后解析HTML结构。但请注意,实际的抓取过程可能因链家网站的反爬策略而变化,你可能需要处理验证码、登录等问题。
首先,请确保已经安装了`requests`和`beautifulsoup4`库(如果未安装,可以使用pip进行安装):
```bash
pip install requests beautifulsoup4
```
接下来,创建一个简单的Python脚本,用于获取页面内容并解析HTML:
```python
import requests
from bs4 import BeautifulSoup
# 链家房源链接
url = 'https://bj.lianjia.com/zufang/'
def get_html(url):
try:
response = requests.get(url, timeout=5) # 设置超时时间防止阻塞
response.raise_for_status() # 检查状态码是否正常
return response.text
except (requests.exceptions.RequestException, ValueError) as e:
print(f"Error occurred while fetching the page: {e}")
return None
def parse_html(html):
soup = BeautifulSoup(html, "html.parser")
# 根据链家网站的HTML结构定位租房信息元素
# 这里假设租房信息包含在class为'list-item'的div标签内
listings = soup.find_all("div", class_="list-item")
for listing in listings:
# 提取并打印必要的信息,比如标题、价格等
title = listing.find("h3").text if listing.h3 else ""
price = listing.find("span", class_='price').text if listing.span and 'price' in listing.span['class'] else ""
print(f"Title: {title}, Price: {price}")
if __name__ == "__main__":
html_content = get_html(url)
if html_content is not None:
parse_html(html_content)
else:
print("Failed to fetch the data.")
```
这个脚本只是一个起点,实际的HTML结构可能会更复杂,可能需要根据链家网站的具体布局调整CSS选择器。此外,如果你需要频繁抓取或大量数据,使用Scrapy这样的框架会更有优势,因为它提供了更强大的爬虫功能,包括设置请求头、代理IP、分布式爬取等。
阅读全文
相关推荐
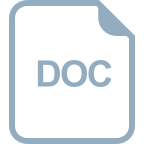















