使用Python处理数据,如何将dataframe中某列等于296,301,440这几个值的数据去除
时间: 2024-10-24 14:17:42 浏览: 14
在Python的Pandas库中,你可以通过条件筛选(filtering)来移除DataFrame中某一列指定值的数据。以下是步骤:
1. 首先,假设你的DataFrame名为`df`,需要检查和过滤的列名是`column_name`。
```python
import pandas as pd
# 假设 df 是你的 DataFrame
```
2. 创建一个布尔索引(Boolean Index),该索引基于特定条件(在这个例子中是`column_name`列的值等于296、301或440):
```python
mask = (df['column_name'] != 296) & (df['column_name'] != 301) & (df['column_name'] != 440)
```
这里 `&` 表示逻辑与操作符,它会同时满足所有条件。
3. 使用这个布尔索引从原始DataFrame中选择出你需要保留的行:
```python
df_filtered = df[mask]
```
现在`df_filtered`就是去除了指定值后的DataFrame。
相关问题
dataframe某列是英文text,怎么提取关键词和其他某列权重,保留权重高的几个词语,举例
您可以使用Python中的nltk库和sklearn库来提取关键词和计算权重。下面是一个简单的示例代码:
```python
import pandas as pd
from sklearn.feature_extraction.text import CountVectorizer
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
# 读取数据
df = pd.read_csv('data.csv')
#除停用词并分词
stop_words = set(stopwords.words('english'))
df['text'] = df['text'].apply(lambda x: [word for word in word_tokenize(x.lower()) if word.isalpha() and word not in stop_words])
# 计算词频
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(df['text'].apply(lambda x: ' '.join(x)))
# 计算TF-IDF权重
from sklearn.feature_extraction.text import TfidfTransformer
transformer = TfidfTransformer()
tfidf = transformer.fit_transform(X)
# 提取权重最高的前n个词
n = 5
top_n = []
for i in range(len(df)):
row = tfidf[i].toarray()[0]
top_n_indices = row.argsort()[-n:][::-1]
top_n_words = [vectorizer.get_feature_names()[i] for i in top_n_indices]
top_n_weights = [row[i] for i in top_n_indices]
top_n.append(list(zip(top_n_words, top_n_weights)))
# 添加到DataFrame中
df['top_n'] = top_n
```
该代码将数据读取为一个DataFrame,然后对每个文本进行分词、去除停用词、计算词频和TF-IDF权重,并提取权重最高的前n个词。最后,将结果添加到DataFrame中的新列```top_n```中。您可以根据需要更改n的值,以提取更多或更少的关键词。
从一个CSV文件中读取URL,然后访问这些URL并从网页中提取表格数据,最后将所有数据合并到一个DataFrame中并保存到一个新的CSV文件中python代码如何写
要从CSV文件中读取URL,访问这些URL并从网页中提取表格数据,最后将数据合并到一个DataFrame中并保存到新的CSV文件,你可以使用Python的几个库,比如`pandas`用于数据处理,`csv`用于读写CSV文件,以及`requests`和`BeautifulSoup`用于网页请求和解析。以下是一个简单的代码示例:
```python
import pandas as pd
import csv
import requests
from bs4 import BeautifulSoup
# 读取CSV文件中的URL
urls_df = pd.read_csv('urls.csv') # 假设CSV文件名为urls.csv,其中只包含URL列
url_list = urls_df['URL'].tolist() # 假设列名是'URL'
# 准备存储数据的列表
data_list = []
# 访问每个URL并从网页中提取表格数据
for url in url_list:
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
tables = soup.find_all('table') # 查找页面中的所有表格
# 假设我们只处理第一个表格
if tables:
table = tables[0]
table_data = []
# 遍历表格中的每一行
for row in table.find_all('tr'):
cols = row.find_all('td')
cols = [ele.text.strip() for ele in cols]
table_data.append([ele for ele in cols if ele]) # 去除空白数据
# 如果表格数据存在,添加到数据列表中
if table_data:
data_list.append(pd.DataFrame(table_data[1:], columns=table_data[0])) # 假设第一行是表头
# 合并所有DataFrame到一个DataFrame中
final_df = pd.concat(data_list, ignore_index=True)
# 保存到新的CSV文件中
final_df.to_csv('merged_data.csv', index=False)
```
在运行上述代码之前,请确保你已经安装了所需的库,可以通过以下命令安装:
```bash
pip install pandas requests beautifulsoup4
```
这里有一些重要的注意事项:
- 确保CSV文件格式正确,且列名与代码中的相匹配。
- 代码中的网页表格解析部分可能需要根据实际的HTML结构进行调整。
- 如果网页内容是动态加载的,可能需要使用像Selenium这样的工具来模拟浏览器行为。
- 网络请求可能会失败,因此考虑添加异常处理机制来增强代码的健壮性。
阅读全文
相关推荐
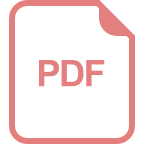
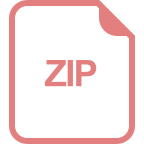
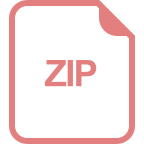
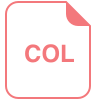
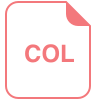
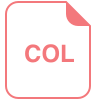
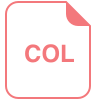
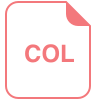
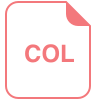






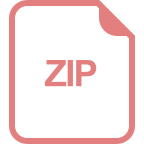
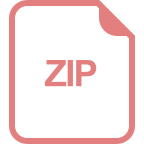
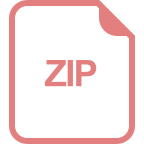