C语言做智能密码强度检测器
时间: 2024-12-21 17:29:46 浏览: 6
C语言可以编写一个简单的智能密码强度检测器,它通常会评估密码的一些关键特征,如长度、包含字符的复杂性(数字、字母大小写、特殊字符)、以及是否满足一定的规则(例如必须包含大写字母、小写字母和数字)。下面是一个简化的示例:
```c
#include <stdio.h>
#include <stdbool.h>
// 定义密码强度级别
enum PasswordStrength {
WEAK, // 密码太短或过于简单
FAIR, // 包含一些基本元素但不复杂
STRONG // 符合复杂性要求
};
// 检测函数
enum PasswordStrength check_password_strength(char *password) {
int num_chars = strlen(password);
bool has_num = false, has_upper = false, has_lower = false;
for (int i = 0; password[i] != '\0'; i++) {
if (isdigit(password[i])) {
has_num = true;
} else if (isupper(password[i])) {
has_upper = true;
} else if (islower(password[i])) {
has_lower = true;
}
}
// 判断强度
if (num_chars < 8 || (!has_num && !has_upper && !has_lower)) {
return WEAK;
} else if (!has_num || !has_upper || !has_lower) {
return FAIR;
} else {
return STRONG;
}
}
int main() {
char password[50];
printf("Enter your password: ");
fgets(password, sizeof(password), stdin); // 防止读取换行符
password[strlen(password) - 1] = '\0'; // 去除末尾的换行符
enum PasswordStrength strength = check_password_strength(password);
switch (strength) {
case WEAK:
printf("Password is weak.\n");
break;
case FAIR:
printf("Password is fair.\n");
break;
case STRONG:
printf("Password is strong.\n");
break;
}
return 0;
}
阅读全文
相关推荐
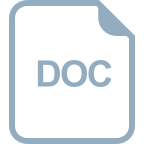
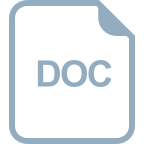
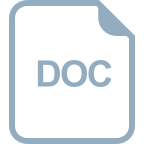
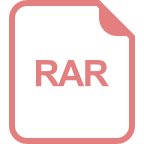
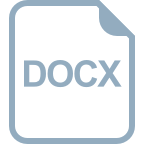
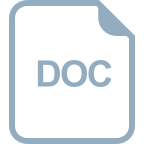
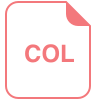
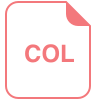
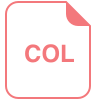
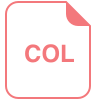
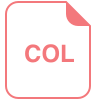
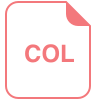
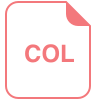
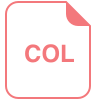
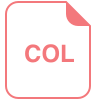
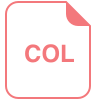
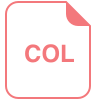
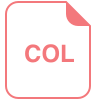