用C语言写销毁顺序表
时间: 2024-09-29 22:04:01 浏览: 18
在C语言中,销毁顺序表(也称为数组或动态数组)通常涉及到释放内存,特别是当你使用动态内存分配(如`malloc()`)创建了这个列表时。以下是一个基本的例子,展示了如何按照元素从后向前的顺序删除并释放元素:
```c
#include <stdio.h>
#include <stdlib.h> // 包含 malloc 和 free
// 定义结构体或数据类型
typedef struct Node {
int data;
struct Node* next; // 指向下一个节点的指针
} Node;
// 插入节点到顺序表尾部
void insert(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) { // 如果内存分配失败,处理错误
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
// 销毁顺序表
void destroyList(Node** head) {
Node* current = *head;
while (current != NULL) {
Node* temp = current;
current = current->next; // 移动当前指针到下一个元素
free(temp); // 释放当前节点的内存
}
*head = NULL; // 更新头指针,表示链表已空
}
int main() {
Node* head = NULL;
// 假设我们插入了一些元素
insert(&head, 1);
insert(&head, 2);
insert(&head, 3);
// 销毁顺序表
destroyList(&head);
return 0;
}
```
在这个例子中,`destroyList` 函数遍历整个顺序表,每次迭代都释放当前节点,然后移动到下一个节点,直到所有节点都被释放。
相关推荐
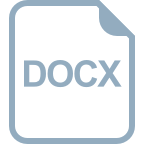
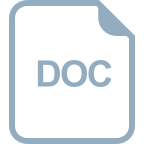
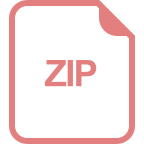














