用C语言实现顺序表的打印,插入,删除,逆置,销毁,初始化
时间: 2024-09-10 16:25:05 浏览: 85
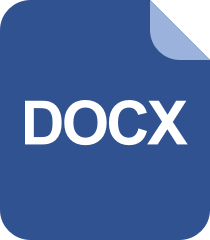
(数据结构C语言版)顺序表和单链表的逆置.docx
在C语言中,我们可以使用数组或动态内存分配的数据结构来实现顺序表(也叫线性表)。这里是一个简单的示例,展示如何操作顺序表的基本功能:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序表节点
typedef struct Node {
int data;
struct Node* next;
} ListNode;
// 初始化顺序表
ListNode* initializeList() {
ListNode* head = (ListNode*)malloc(sizeof(ListNode));
if (!head) {
printf("Memory allocation failed.\n");
return NULL;
}
head->data = 0; // 初始化第一个元素为空
head->next = NULL;
return head;
}
// 打印顺序表
void printList(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// 插入元素
void insertNode(ListNode** head, int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
// 删除元素
void deleteNode(ListNode** head, int value) {
if (*head == NULL) {
return;
}
if ((*head)->data == value) {
ListNode* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
ListNode* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
ListNode* temp = current->next;
current->next = current->next->next;
free(temp);
}
}
// 逆置顺序表
void reverseList(ListNode** head) {
ListNode* prev = NULL;
ListNode* current = *head;
ListNode* next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
*head = prev;
}
// 销毁顺序表
void destroyList(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
ListNode* temp = current;
current = current->next;
free(temp);
}
}
int main() {
ListNode* list = initializeList();
// 使用insertNode等函数进行操作...
printList(list); // 打印当前列表
// ...
destroyList(list); // 销毁列表前记得调用
return 0;
}
```
阅读全文
相关推荐
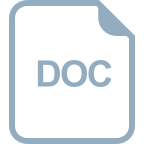
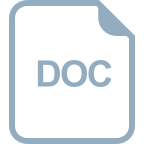
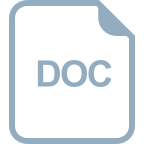
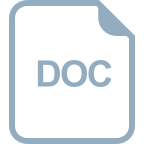
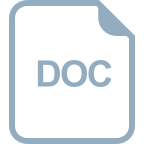
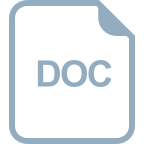
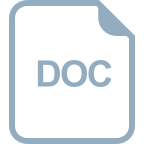
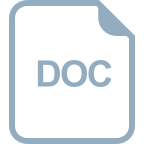
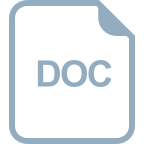
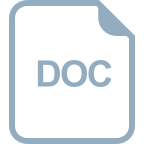
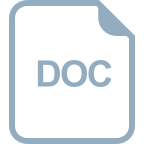
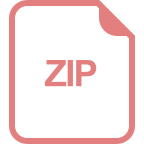
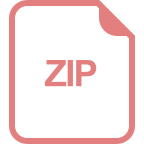
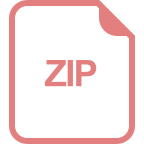
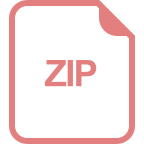