document.addEventListener('DOMContentLoaded', function() { const form = document.getElementById('registration-form'); form.addEventListener('submit', function (event) { event.preventDefault(); // 阻止表单提交 const name = document.getElementById('name').value; const password = document.getElementById('password').value; const email = document.getElementById('email').value; console.log('注册信息:'); console.log('用户名:', name); console.log('密码:', password); console.log('邮箱:', email); }); }); const express = require('express'); const bodyParser = require('body-parser'); const mssql = require('mssql'); const app = express(); app.use(bodyParser.urlencoded({ extended: false })); // 创建与数据库的连接 const connection = mssql.createConnection({ server: '192.168.10.223', user: 'sa', password: 'Servo01', database: 'demo' }); // 连接数据库 connection.connect(function (err) { if (err) { console.error('Error connecting to database:', err); return; } console.log('Connected to database!'); }); // 处理注册页面的 POST 请求 app.post('/', function (req, res) { const name = req.body.name; const password = req.body.password; const email = req.body.email; console.log('注册信息:'); console.log('用户名:', name); console.log('密码:', password); console.log('邮箱:', email); // 将数据保存到数据库 const query = `INSERT INTO username (name, password, email) VALUES ('${name}','${password}','${email}')`; const request = new mssql.Request(); request.query(query, function (err, request) { if (err) { console.error('Error saving data to database:', err); return; } console.log('数据已保存到数据库!'); }); res.send('注册成功!'); }); app.listen(3000, function () { console.log('Server is running on port 3000'); });
时间: 2024-02-04 17:02:48 浏览: 127
根据你提供的代码,我注意到以下问题:
1. SQL 注入攻击:在构建 SQL 查询语句时,你直接使用了用户输入的数据拼接成字符串。这种做法存在安全风险,可能导致 SQL 注入攻击。为了避免这个问题,强烈建议使用参数化查询或预编译语句来处理用户输入的数据,而不是直接拼接字符串。可以使用 `mssql` 模块提供的参数化查询功能来实现这一点。
2. 请求对象的创建:在处理注册页面的 POST 请求时,你在创建请求对象时使用了错误的方法。对于 `mssql` 模块,应该使用 `new mssql.Request()` 来创建请求对象,而不是 `new Request()`。
3. 数据库操作错误处理:在数据库操作中,没有对错误进行适当处理。当插入数据失败时,应该捕获错误并进行适当的错误处理,例如打印错误信息或返回错误状态给客户端。
请根据上述问题进行修正,并仔细检查代码是否存在其他潜在问题。如果问题还是无法解决,请提供详细的错误信息以便更好地帮助你解决问题。
相关问题
// 监听标签页切换事件 document.addEventListener('DOMContentLoaded', function() { const tabs = document.querySelectorAll('.switch-bar a-tab-pane'); tabs.forEach(tab => { tab.addEventListener('click', function() { // 判断switch-bar是否固定在顶部 const switchBar = document.getElementById('switchBar'); const isSticky = switchBar.getBoundingClientRect().top === 0; // 如果固定在顶部,则让页面回跳到顶部 if (isSticky) { window.scrollTo({ top: 0, behavior: 'smooth' }); } }); }); });这个放在哪里?
这段代码可以放在你的JavaScript文件中的任何位置,只要确保它在DOM加载完成后执行即可。以下是几个常见的放置位置:
1. 放在`<script>`标签中:你可以将这段代码直接放在`<script>`标签中,并将该标签放在HTML文件的`<body>`标签的末尾。例如:
```html
<body>
<!-- 页面内容 -->
<script>
document.addEventListener('DOMContentLoaded', function() {
// 监听标签页切换事件的代码
});
</script>
</body>
```
2. 外部JavaScript文件:如果你的JavaScript代码较长或者需要在多个页面中使用,可以将代码保存为外部的JavaScript文件,并在HTML文件中使用`<script>`标签引入该文件。例如:
```html
<body>
<!-- 页面内容 -->
<script src="path/to/your/script.js"></script>
</body>
```
在`script.js`文件中,放置监听标签页切换事件的代码。
无论你选择哪种方式,确保代码在DOM加载完成后执行,以便正确地绑定事件监听器。
document.addEventListener('DOMContentLoaded', function() { //获取按钮和输入框的元素 const generateBtn = document.getElementById('10'); const phoneInput = document.getElementById('7'); const verifyInput = document.getElementById('8'); const submitBtn = document.getElementById('submit-btn'); let randomNum = null; //存储随机数的变量 //生成随机数并弹窗显示 generateBtn.addEventListener('click', function(event) { event.preventDefault(); //如果手机号不满足要求,则弹出手机号错误的提示框 if (!validatePhone()) { alert('手机号错误'); return; } //如果随机数还未生成,则生成新的随机数 if (randomNum === null) { randomNum = Math.floor(Math.random() * 10000); } //弹窗显示随机数 alert('验证码:' + randomNum); }); //提交按钮点击事件监听器 submitBtn.addEventListener('click', function(event) { //获取存储的随机数 const storedRandomNum = randomNum; //获取用户输入的验证码和手机号 const userEnteredNum = parseInt(verifyInput.value); const phoneValue = phoneInput.value; //检查是否已生成随机数 if (storedRandomNum === null) { event.preventDefault(); } else if (userEnteredNum !== storedRandomNum) { //检查验证码是否正确 event.preventDefault(); alert('验证码错误'); } else { //验证码正确,提交表单 //这里可以添加其他逻辑或调用其他函数来处理表单提交 } }); //手机号验证函数 function validatePhone() { const phoneValue = phoneInput.value; //检查手机号是否为11个数字 return /^\d{11}$/.test(phoneValue); } });
刚进入界面时不应该弹出"手机号错误"的弹窗,因为此时用户还没有进行任何操作。可能的原因是在页面加载完成后,自动触发了生成随机数按钮的点击事件。
请检查页面加载完成后是否存在其他代码或事件触发了生成随机数按钮的点击事件。可以通过在生成随机数按钮的点击事件处理函数中添加 console.log() 或者 alert() 来进行调试,查看是否在页面加载完成时就执行了生成随机数按钮的点击事件。
另外,也要确保生成随机数按钮的相关元素(如id为 '10')存在于页面中,并且与对应的按钮元素匹配。
阅读全文
相关推荐
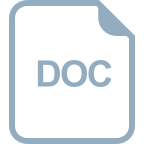
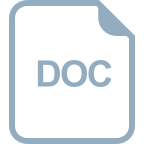
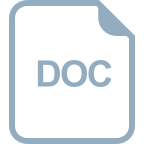








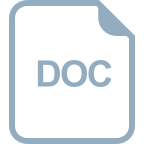
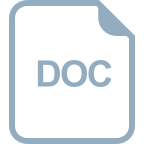
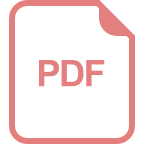
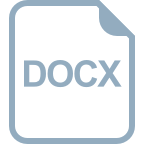
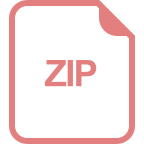