C语言定义一个成绩数据链表,读入相应的成绩数据来完成学生成绩信息表创建,并实现成绩的输出,查找,插入,删除
时间: 2024-10-16 19:08:16 浏览: 18
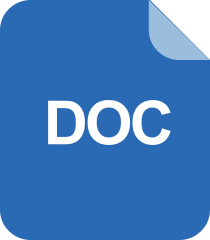
数据结构之学生成绩管理系统.doc

在C语言中,我们可以使用结构体(struct)来定义一个成绩数据结构,并结合数组或者动态内存分配(如指针和链接列表)来实现一个成绩数据链表。首先,我们定义一个表示学生和成绩的结构体:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生和成绩的结构体
typedef struct {
char name[50];
int score;
} Student;
// 创建链表头节点
typedef struct Node {
Student data;
struct Node* next;
} Node;
// 链表操作函数声明
Node* createList();
void displayList(Node* head);
Student* searchByName(Node* head, const char* name);
void insertStudent(Node** head, const char* name, int score);
void deleteStudent(Node** head, const char* name);
int main() {
// 初始化链表
Node* head = createList();
// 插入、显示、查找和删除操作...
// ...
return 0;
}
// 创建一个新的链表
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node));
if (!head) {
printf("Memory allocation failed.\n");
exit(1);
}
head->data.name[0] = '\0';
head->next = NULL;
return head;
}
// 显示链表中的所有学生信息
void displayList(Node* head) {
while (head != NULL) {
printf("%s: %d\n", head->data.name, head->data.score);
head = head->next;
}
}
// 搜索指定名字的学生并返回其信息
Student* searchByName(Node* head, const char* name) {
while (head != NULL && strcmp(head->data.name, name) != 0) {
head = head->next;
}
return head ? &head->data : NULL;
}
// 插入新学生到链表中
void insertStudent(Node** head, const char* name, int score) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed for new node.\n");
return;
}
strcpy(newNode->data.name, name);
newNode->data.score = score;
newNode->next = *head;
*head = newNode;
}
// 删除指定名字的学生
void deleteStudent(Node** head, const char* name) {
Node* current = *head;
Node* prev = NULL;
while (current != NULL && strcmp(current->data.name, name) != 0) {
prev = current;
current = current->next;
}
if (current == NULL) {
printf("Student not found.\n");
return;
}
if (prev == NULL) {
*head = current->next; // 头部删除
} else {
prev->next = current->next;
}
free(current); // 释放已删除节点的内存
}
```
在这个例子中,我们实现了创建链表、显示链表、搜索特定学生、插入学生以及删除学生的基本功能。你可以根据实际需求在`main()`函数中调用这些操作。
阅读全文
相关推荐
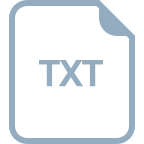
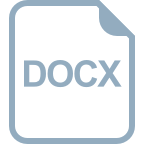













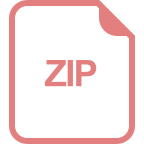
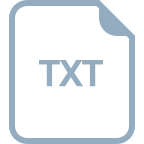
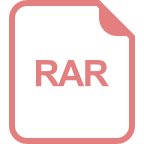