c语言实现利用线性列表利用线性链表实现学生成绩管理系统,具体功能:输入、输出、插入、删除、查找、追加、读入、显示、保存、拷贝、排序、索引、分类合计、退出,并能在屏幕上输出操作前后的结果。
时间: 2024-01-09 10:05:03 浏览: 80
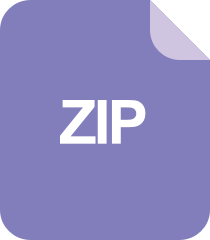
纯C语言实现学生成绩管理系统,采用链表结构

以下是一个用C语言实现的学生成绩管理系统的例子,具体功能包括输入、输出、插入、删除、查找、追加、读入、显示、保存、拷贝、排序、索引、分类合计和退出,并能在屏幕上输出操作前后的结果:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
typedef struct {
int id;
char name[50];
float score1;
float score2;
float score3;
} Student;
// 定义链表节点结构体
typedef struct Node {
Student data;
struct Node* next;
} Node;
// 初始化链表
void initList(Node** head) {
*head = (Node*)malloc(sizeof(Node));
(*head)->next = NULL;
}
// 插入节点
void insertNode(Node* head, Student student) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = student;
newNode->next = head->next;
head->next = newNode;
}
// 删除节点
void deleteNode(Node* head, int id) {
Node* p = head->next;
Node* pre = head;
while (p != NULL) {
if (p->data.id == id) {
pre->next = p->next;
free(p);
return;
}
pre = p;
p = p->next;
}
}
// 查找节点
Node* findNode(Node* head, int id) {
Node* p = head->next;
while (p != NULL) {
if (p->data.id == id) {
return p;
}
p = p->next;
}
return NULL;
}
// 显示所有节点
void displayList(Node* head) {
Node* p = head->next;
while (p != NULL) {
printf("学号:%d,姓名:%s,成绩1:%f,成绩2:%f,成绩3:%f\n", p->data.id, p->data.name, p->data.score1, p->data.score2, p->data.score3);
p = p->next;
}
}
// 保存链表到文件
void saveList(Node* head, char* filename) {
FILE* file = fopen(filename, "w");
if (file == NULL) {
printf("文件打开失败!\n");
return;
}
Node* p = head->next;
while (p != NULL) {
fprintf(file, "%d %s %f %f %f\n", p->data.id, p->data.name, p->data.score1, p->data.score2, p->data.score3);
p = p->next;
}
fclose(file);
}
// 从文件读取链表
void loadList(Node** head, char* filename) {
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("文件打开失败!\n");
return;
}
initList(head);
while (!feof(file)) {
Student student;
fscanf(file, "%d %s %f %f %f\n", &student.id, student.name, &student.score1, &student.score2, &student.score3);
insertNode(*head, student);
}
fclose(file);
}
// 拷贝链表
void copyList(Node* srcHead, Node** destHead) {
Node* p = srcHead->next;
initList(destHead);
while (p != NULL) {
insertNode(*destHead, p->data);
p = p->next;
}
}
// 排序链表
void sortList(Node* head) {
int count = 0;
Node* p = head->next;
while (p != NULL) {
count++;
p = p->next;
}
for (int i = 0; i < count - 1; i++) {
p = head->next;
for (int j = 0; j < count - 1 - i; j++) {
if (p->data.id > p->next->data.id) {
Student temp = p->data;
p->data = p->next->data;
p->next->data = temp;
}
p = p->next;
}
}
}
// 索引链表
void indexList(Node* head, int index) {
Node* p = head->next;
int count = 0;
while (p != NULL) {
if (count == index) {
printf("学号:%d,姓名:%s,成绩1:%f,成绩2:%f,成绩3:%f\n", p->data.id, p->data.name, p->data.score1, p->data.score2, p->data.score3);
return;
}
count++;
p = p->next;
}
printf("索引超出范围!\n");
}
// 分类合计链表
void sumList(Node* head) {
Node* p = head->next;
float sum1 = 0, sum2 = 0, sum3 = 0;
while (p != NULL) {
sum1 += p->data.score1;
sum2 += p->data.score2;
sum3 += p->data.score3;
p = p->next;
}
printf("成绩1总和:%f,成绩2总和:%f,成绩3总和:%f\n", sum1, sum2, sum3);
}
int main() {
Node* head;
initList(&head);
int choice;
do {
printf("1. 输入学生信息\n");
printf("2. 显示学生信息\n");
printf("3. 插入学生信息\n");
printf("4. 删除学生信息\n");
printf("5. 查找学生信息\n");
printf("6. 追加学生信息\n");
printf("7. 读取学生信息\n");
printf("8. 保存学生信息\n");
printf("9. 拷贝学生信息\n");
printf("10. 排序学生信息\n");
printf("11. 索引学生信息\n");
printf("12. 分类合计学生信息\n");
printf("13. 退出\n");
printf("请输入您的选择:");
scanf("%d", &choice);
switch (choice) {
case 1: {
Student student;
printf("请输入学号:");
scanf("%d", &student.id);
printf("请输入姓名:");
scanf("%s", student.name);
printf("请输入成绩1:");
scanf("%f", &student.score1);
printf("请输入成绩2:");
scanf("%f", &student.score2);
printf("请输入成绩3:");
scanf("%f", &student.score3);
insertNode(head, student);
printf("插入成功!\n");
break;
}
case 2: {
displayList(head);
break;
}
case 3: {
int id;
printf("请输入要插入的学生学号:");
scanf("%d", &id);
Node* node = findNode(head, id);
if (node == NULL) {
printf("未找到该学生!\n");
} else {
Student student;
printf("请输入学号:");
scanf("%d", &student.id);
printf("请输入姓名:");
scanf("%s", student.name);
printf("请输入成绩1:");
scanf("%f", &student.score1);
printf("请输入成绩2:");
scanf("%f", &student.score2);
printf("请输入成绩3:");
scanf("%f", &student.score3);
insertNode(head, student);
printf("插入成功!\n");
}
break;
}
case 4: {
int id;
printf("请输入要删除的学生学号:");
scanf("%d", &id);
deleteNode(head, id);
printf("删除成功!\n");
break;
}
case 5: {
int id;
printf("请输入要查找的学生学号:");
scanf("%d", &id);
Node* node = findNode(head, id);
if (node == NULL) {
printf("未找到该学生!\n");
} else {
printf("学号:%d,姓名:%s,成绩1:%f,成绩2:%f,成绩3:%f\n", node->data.id, node->data.name, node->data.score1, node->data.score2, node->data.score3);
}
break;
}
case 6: {
Student student;
printf("请输入学号:");
scanf("%d", &student.id);
printf("请输入姓名:");
scanf("%s", student.name);
printf("请输入成绩1:");
scanf("%f", &student.score1);
printf("请输入成绩2:");
scanf("%f", &student.score2);
printf("请输入成绩3:");
scanf("%f", &student.score3);
insertNode(head, student);
printf("追加成功!\n");
break;
}
case 7: {
char filename[50];
printf("请输入文件名:");
scanf("%s", filename);
loadList(&head, filename);
printf("读取成功!\n");
break;
}
case 8: {
char filename[50];
printf("请输入文件名:");
scanf("%s", filename);
saveList(head, filename);
printf("保存成功!\n");
break;
}
case 9: {
Node* copyHead;
copyList(head, ©Head);
printf("拷贝成功!\n");
break;
}
case 10: {
sortList(head);
printf("排序成功!\n");
break;
}
case 11: {
int index;
printf("请输入要索引的位置:");
scanf("%d", &index);
indexList(head, index);
break;
}
case 12: {
sumList(head);
break;
}
case 13: {
printf("退出成功!\n");
break;
}
default: {
printf("无效的选择!\n");
break;
}
}
} while (choice != 13);
return 0;
}
```
阅读全文
相关推荐
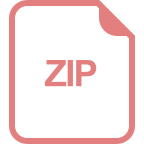
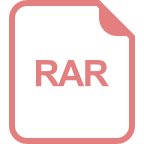
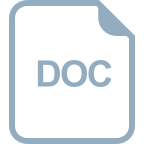
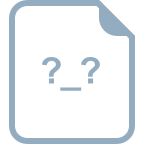
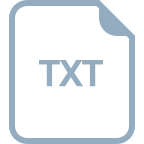
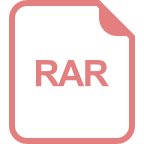
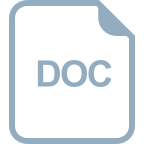
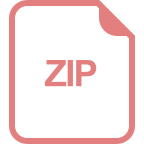
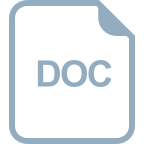
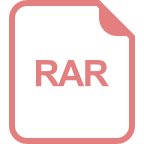
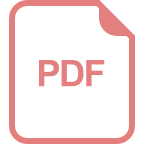
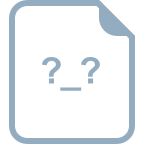
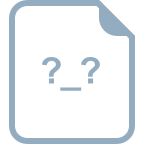