用c语言写一段代码,要求使用顺序表的操作(新建,消除,定位,查找,插入,删除,修改,输出,判断空,满。
时间: 2024-09-18 10:01:03 浏览: 18
在C语言中,我们可以使用数组或结构体来实现简单的顺序表操作。这里是一个基本的例子,我们创建一个`Node`结构体作为元素,并包含一个指向下一个节点的指针。下面是一些常用的操作:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 新建顺序表
Node* create_list() {
return (Node*)malloc(sizeof(Node));
}
// 检查列表是否为空
int is_empty(Node* head) {
return head == NULL;
}
// 插入数据到列表尾部
void insert(Node** head, int value) {
Node* newNode = create_list();
if (is_empty(*head)) {
newNode->next = *head;
*head = newNode;
} else {
Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
newNode->data = value;
newNode->next = NULL;
}
}
// 查找特定值并返回其位置(从0开始)
int find_position(Node* head, int value) {
Node* temp = head;
int position = 0;
while (temp != NULL && temp->data != value) {
temp = temp->next;
position++;
}
if (temp == NULL) {
return -1; // 如果未找到则返回-1
}
return position;
}
// 删除指定位置的数据
void delete(Node** head, int position) {
if (is_empty(*head)) return;
if (position == 0) { // 删除头部
Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Node* current = *head;
for (int i = 0; i < position - 1; i++) {
current = current->next;
}
if (current->next == NULL) return; // 不存在该位置,忽略
Node* temp = current->next;
current->next = temp->next;
free(temp);
}
// 修改指定位置的数据
void modify(Node** head, int position, int new_value) {
if (position >= 0 && !is_empty(*head)) {
Node* temp = *head;
for (int i = 0; i < position; i++) {
temp = temp->next;
}
temp->data = new_value;
} else {
printf("Invalid position.\n");
}
}
// 输出整个列表
void print_list(Node* head) {
if (is_empty(head)) {
printf("Empty list.\n");
} else {
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
}
int main() {
Node* list = create_list();
// 使用示例
insert(&list, 5);
insert(&list, 10);
insert(&list, 15);
print_list(list); // 输出: 5 10 15
int index = find_position(list, 10);
if (index != -1)
printf("Found at position %d\n", index); // 输出: Found at position 1
delete(&list, 1);
print_list(list); // 输出: 5 15
modify(&list, 0, 7);
print_list(list); // 输出: 7 15
return 0;
}
```
相关推荐
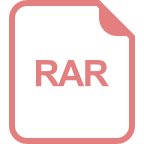
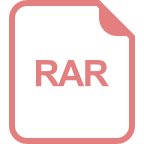















