C# 枚举类型 根据
时间: 2024-08-15 14:07:14 浏览: 34
枚举类型(Enum)在C#中是一种特殊的数据类型,用于表示一组固定的、有命名的整数值集合。这种类型能够帮助提高代码的清晰度和安全性,因为它限制了变量只能取预定义的一组常数值之一。
### 定义枚举
在C#中,你可以通过以下语法定义枚举:
```csharp
enum Color
{
Red,
Green,
Blue,
Yellow
}
```
在这个例子中,我们定义了一个名为`Color`的枚举类型,它包含了四种颜色:Red(红色)、Green(绿色)、Blue(蓝色)和Yellow(黄色)。每个枚举成员都对应一个整数值,顺序递增。第一个成员默认值为0,第二个成员为1,依此类推。
### 使用枚举
一旦定义了枚举类型,就可以像使用其他数据类型一样在代码中使用它:
```csharp
int colorIndex;
colorIndex = (int)Color.Red; // 将颜色Red转换为其对应的整数值
Console.WriteLine(colorIndex); // 输出: 0
Color userChoice = Color.Blue; // 初始化用户的选择为蓝色
if (userChoice == Color.Green)
{
Console.WriteLine("You chose green!");
}
else if (userChoice == Color.Blue)
{
Console.WriteLine("You chose blue!");
}
else
{
Console.WriteLine("Invalid choice.");
}
```
### 扩展枚举功能
除了基本的功能外,C#还允许你给枚举添加属性和事件,以及静态方法和构造函数。这使得枚举不仅仅是简单的值集合,还能提供额外的结构化信息和服务。例如:
```csharp
enum Season : int
{
Winter,
Spring,
Summer,
Autumn,
// 添加描述性的名字
[Description("冬季")]
冬季,
[Description("春季")]
春季,
[Description("夏季")]
夏季,
[Description("秋季")]
秋季,
}
class Program
{
static void Main()
{
string description = Season.Describe(Season.Winter);
Console.WriteLine(description); // 输出: 冬季
}
public static string Describe(Season season)
{
return ((season).GetType().GetMember(season.ToString()).GetCustomAttribute<DescriptionAttribute>()?.Description ?? "");
}
}
```
在这个例子中,我们使用`[Description]`属性来提供每个季节的英文名及其对应的中文翻译。此外,我们定义了一个静态方法`Describe`来获取枚举成员的描述。
### 相关问题:
1. 在哪种情况下最适合使用枚举类型?
2. 如何在C#中为枚举成员添加自定义属性或事件?
3. 枚举是否支持继承或其他多态特性?
相关推荐
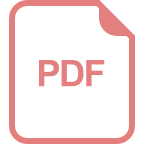
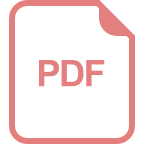
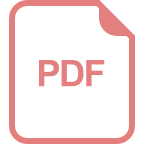














