vue2上传csv文件到后端,展示到表格中
时间: 2024-04-14 13:28:37 浏览: 14
对于Vue 2,可以使用类似的方法来实现上传CSV文件并将其展示在表格中的功能。下面是一个示例代码:
前端部分(Vue组件):
```vue
<template>
<div>
<input type="file" @change="uploadFile" />
<table>
<thead>
<tr>
<th v-for="header in headers" :key="header">{{ header }}</th>
</tr>
</thead>
<tbody>
<tr v-for="data in tableData" :key="data.id">
<td v-for="header in headers" :key="header">{{ data[header] }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
headers: [],
tableData: []
};
},
methods: {
uploadFile(event) {
const file = event.target.files[0];
const formData = new FormData();
formData.append('file', file);
fetch('/api/upload', {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(jsonData => {
this.headers = Object.keys(jsonData[0]);
this.tableData = jsonData;
})
.catch(error => {
console.error(error);
});
}
}
};
</script>
```
后端部分(Node.js示例,使用Express框架):
```javascript
const express = require('express');
const csv = require('csv-parser');
const fs = require('fs');
const app = express();
app.use(express.json());
app.post('/api/upload', (req, res) => {
const results = [];
req.busboy.on('file', (fieldname, file, filename) => {
file.pipe(csv())
.on('data', (data) => results.push(data))
.on('end', () => {
res.json(results);
});
});
req.pipe(req.busboy);
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
请注意,这只是一个简单的示例,你可能需要根据实际需求进行一些修改和优化。另外,确保在后端使用合适的中间件(如`connect-busboy`)来处理文件上传。
相关推荐
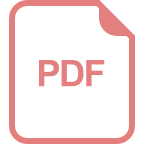
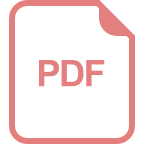
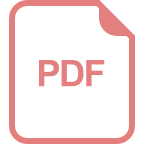
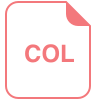
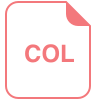
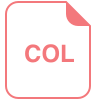
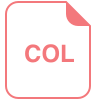
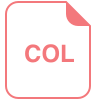









