vue3子组件调用父组件的函数,并获取返回值在子组件进行赋值
时间: 2023-11-29 14:43:57 浏览: 266
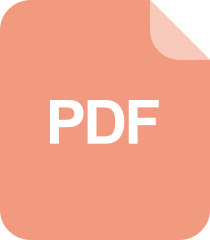
Vue子组件向父组件通信与父组件调用子组件中的方法

在Vue3中,子组件可以通过`$emit`方法向父组件发送事件,父组件可以通过`v-on`指令监听子组件的事件并调用相应的方法。如果需要在子组件中获取父组件方法的返回值并进行赋值,可以使用`async/await`结合`$emit`和`v-on`来实现。
具体步骤如下:
1. 在父组件中定义一个异步方法,并在该方法中调用需要执行的函数并返回结果。
2. 在子组件中通过`$emit`方法向父组件发送事件,并将父组件的方法名和参数传递给父组件。
3. 在父组件中通过`v-on`指令监听子组件的事件,并在事件处理函数中调用相应的方法并将结果通过`$emit`方法返回给子组件。
4. 在子组件中通过`await`关键字等待父组件返回的结果,并将结果赋值给子组件的变量。
代码示例:
父组件:
```html
<template>
<div>
<child-component @get-result="getResult"></child-component>
<p>父组件中的结果:{{ result }}</p>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue'
export default {
components: {
ChildComponent
},
methods: {
async getResult() {
// 调用需要执行的函数并返回结果
const result = await someFunction()
// 将结果通过$emit方法返回给子组件
this.$emit('set-result', result)
}
},
data() {
return {
result: ''
}
}
}
</script>
```
子组件:
```html
<template>
<div>
<button @click="getResult">获取结果</button>
<p>子组件中的结果:{{ result }}</p>
</div>
</template>
<script>
export default {
methods: {
getResult() {
// 向父组件发送事件,并将父组件的方法名和参数传递给父组件
this.$emit('get-result')
}
},
data() {
return {
result: ''
}
},
mounted() {
// 监听父组件通过$emit方法返回的结果,并将结果赋值给子组件的变量
this.$on('set-result', result => {
this.result = result
})
}
}
</script>
```
阅读全文
相关推荐
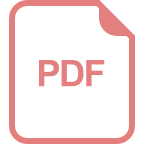
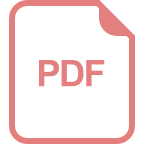















