用Java语言编写一个非连续存储的栈,并实现入栈出栈等操作
时间: 2024-12-19 12:15:38 浏览: 6
在Java中,可以使用数组或者链表(LinkedList)来创建一个非连续存储的栈。这里我们使用链表作为示例,因为链表元素的位置不是固定的,这与非连续存储的概念相吻合。下面是一个简单的链表栈实现:
```java
public class NonContiguousStack {
private Node top; // 栈顶节点
// 结构体Node
static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// 入栈操作 - push
public void push(int item) {
Node newNode = new Node(item);
if (top == null) {
top = newNode;
} else {
newNode.next = top;
top = newNode;
}
}
// 出栈操作 - pop
public int pop() {
if (isEmpty()) {
throw new EmptyStackException();
}
int poppedData = top.data;
top = top.next;
return poppedData;
}
// 查看栈顶元素 - peek
public int peek() {
if (isEmpty()) {
throw new EmptyStackException();
}
return top.data;
}
// 判断栈是否为空
public boolean isEmpty() {
return top == null;
}
}
```
在这个实现中,`push()`方法将新的元素添加到链表头部,`pop()`方法删除并返回栈顶元素,`peek()`则是查看栈顶元素而不实际移除它,而`isEmpty()`用于检查栈是否为空。
阅读全文
相关推荐
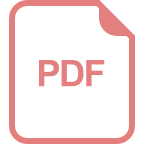
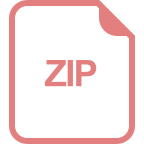
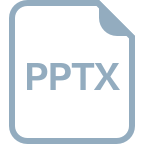



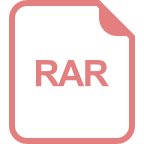





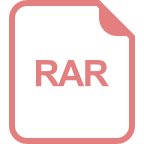
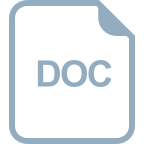



