问题描述:对于给定的一个表达式,表达式中可以包括常数、算术运行符(包括:“+”、“-”、“*”、“/”、“%”(求余运算)、“^”(乘幂运算))和括号,编写程序计算表达式的值。 根据上述问题描述编写程序
时间: 2024-06-14 14:03:51 浏览: 184
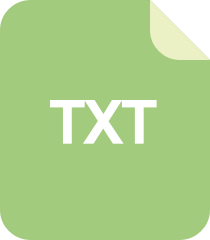
带括号的算术表达式计算程序

根据给定的问题描述,可以使用栈来计算表达式的值。具体步骤如下:
1. 创建两个栈,一个用于存储操作数(operand_stack),一个用于存储运算符(operator_stack)。
2. 遍历表达式中的每个字符:
- 如果字符是数字,则将其转换为整数并将其压入操作数栈。
- 如果字符是左括号,则将其压入运算符栈。
- 如果字符是右括号,则执行以下操作:
- 从运算符栈中弹出运算符,直到遇到左括号。
- 从操作数栈中弹出两个操作数,执行相应的运算,并将结果压入操作数栈。
- 如果字符是运算符,则执行以下操作:
- 如果运算符栈为空,或者栈顶运算符是左括号,则将运算符压入运算符栈。
- 否则,如果当前运算符的优先级小于或等于栈顶运算符的优先级,则从运算符栈中弹出运算符,从操作数栈中弹出两个操作数,执行相应的运算,并将结果压入操作数栈。
- 最后,将当前运算符压入运算符栈。
3. 当表达式遍历完毕后,依次从运算符栈中弹出运算符,从操作数栈中弹出两个操作数,执行相应的运算,并将结果压入操作数栈。
4. 最终,操作数栈中的唯一元素即为表达式的最终值。
下面是一个示例代码,演示如何计算给定表达式的值:
```python
def calculate_expression(expression):
# 定义运算符的优先级
precedence = {'+': 1, '-': 1, '*': 2, '/': 2, '%': 2, '^': 3}
# 定义操作数栈和运算符栈
operand_stack = []
operator_stack = []
# 遍历表达式中的每个字符
for char in expression:
if char.isdigit():
operand_stack.append(int(char))
elif char == '(':
operator_stack.append(char)
elif char == ')':
while operator_stack[-1] != '(':
operator = operator_stack.pop()
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
result = perform_operation(operand1, operand2, operator)
operand_stack.append(result)
operator_stack.pop() # 弹出左括号
elif char in precedence:
while operator_stack and operator_stack[-1] != '(' and precedence[char] <= precedence[operator_stack[-1]]:
operator = operator_stack.pop()
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
result = perform_operation(operand1, operand2, operator)
operand_stack.append(result)
operator_stack.append(char)
# 处理剩余的运算符
while operator_stack:
operator = operator_stack.pop()
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
result = perform_operation(operand1, operand2, operator)
operand_stack.append(result)
return operand_stack[0]
def perform_operation(operand1, operand2, operator):
if operator == '+':
return operand1 + operand2
elif operator == '-':
return operand1 - operand2
elif operator == '*':
return operand1 * operand2
elif operator == '/':
return operand1 // operand2
elif operator == '%':
return operand1 % operand2
elif operator == '^':
return operand1 ** operand2
expression = input("请输入表达式:")
result = calculate_expression(expression)
print("表达式的值为:", result)
```
阅读全文
相关推荐




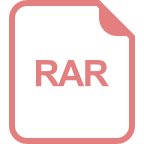
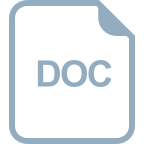
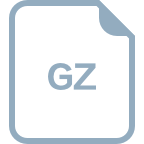
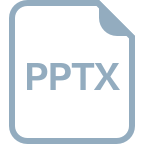
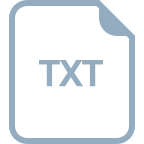
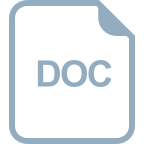
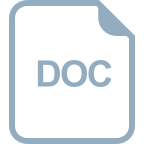
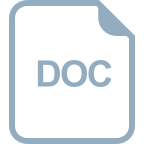
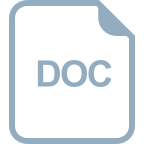
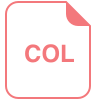
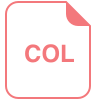
