Python3 的流程控制语句代码示例
时间: 2024-06-02 18:06:57 浏览: 16
当你编写程序时,你需要根据不同的情况执行不同的操作。流程控制语句就是用来控制程序的执行流程,使程序按照你的意愿执行。
以下是Python3中常用的流程控制语句及代码示例:
1. if语句:如果条件为真,执行一段代码块。
```python
x = 10
if x > 5:
print("x is greater than 5")
```
2. if-else语句:如果条件为真,执行第一个代码块;如果条件为假,执行第二个代码块。
```python
x = 3
if x > 5:
print("x is greater than 5")
else:
print("x is less than or equal to 5")
```
3. if-elif-else语句:如果第一个条件为真,执行第一个代码块;否则,如果第二个条件为真,执行第二个代码块;以此类推。如果所有条件都为假,则执行最后一个代码块。
```python
x = 3
if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than 5")
```
4. for循环:遍历一个序列或者其他可迭代对象,执行一段代码块。
```python
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
```
5. while循环:只要条件为真,就一直执行一段代码块。
```python
i = 1
while i < 6:
print(i)
i += 1
```
6. break语句:用于跳出当前循环。
```python
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
if fruit == "banana":
break
print(fruit)
```
7. continue语句:用于跳过当前循环中的一个迭代。
```python
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
if fruit == "banana":
continue
print(fruit)
```
相关推荐
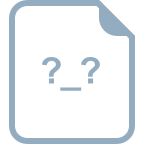
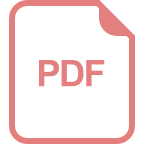














