qt中QGraphicsScene中添加一个qpushbutton;应该如何删除这个qpushbutton
时间: 2024-09-06 18:04:21 浏览: 80
在Qt框架中,如果你在QGraphicsScene中添加了一个QGraphicsItem的子类,比如QPushButton,要删除这个按钮,你需要执行以下步骤:
1. 使用QGraphicsScene的`items()`或`itemsBoundingRect()`方法来检索场景中的项目列表。
2. 遍历这个列表,找到你想要删除的QPushButton对象。
3. 调用QGraphicsScene的`removeItem()`方法,将这个按钮对象从场景中移除。
4. 最后,删除这个按钮对象。由于按钮已经被从场景中移除,这将从内存中彻底删除该对象。
下面是一个简单的代码示例,展示了如何在Qt中从QGraphicsScene中删除一个QPushButton:
```cpp
// 假设你已经有一个名为button的QPushButton指针和一个QGraphicsScene对象scene
QGraphicsItem* item = scene.items().value(0); // 获取场景中的第一个项目,这里假设你已知button是第一个项目
QGraphics.setSceneRect(itemsBoundingRect()); // 设置场景的边界矩形
if (item && item == button) {
scene.removeItem(item); // 从场景中移除按钮
delete item; // 从内存中删除按钮
}
```
请确保在删除graphics item时,场景中不再有指向它的指针,否则可能会引起野指针访问的问题。
相关问题
QPushButton 怎么添加到指定的QGraphicsScene中
要将QPushButton添加到指定的QGraphicsScene中,可以按照以下步骤操作:
1. 创建一个QGraphicsProxyWidget对象,并将QPushButton作为参数传递给它。
2. 将QGraphicsProxyWidget对象添加到QGraphicsScene中。
下面是示例代码:
```python
from PyQt5.QtWidgets import QGraphicsScene, QGraphicsView, QPushButton, QGraphicsProxyWidget
from PyQt5.QtCore import Qt
# 创建QPushButton
button = QPushButton("Click me!")
# 创建QGraphicsScene
scene = QGraphicsScene()
# 创建QGraphicsProxyWidget
proxy = QGraphicsProxyWidget()
proxy.setWidget(button)
proxy.setFlag(QGraphicsItem.ItemIsMovable)
# 将QGraphicsProxyWidget添加到QGraphicsScene中
scene.addItem(proxy)
# 创建QGraphicsView并将QGraphicsScene设置为其场景
view = QGraphicsView(scene)
# 显示视图
view.show()
```
在上述代码中,我们首先创建了QPushButton对象,然后创建了QGraphicsScene对象。接着,我们创建了一个QGraphicsProxyWidget对象,并将QPushButton对象作为参数传递给它,然后将其添加到QGraphicsScene中。最后,我们创建了一个QGraphicsView对象,并将QGraphicsScene设置为其场景,然后显示了视图。
注意,我们还设置了QGraphicsProxyWidget的标志为ItemIsMovable,以使其能够在QGraphicsScene中移动。
QGraphicsScene显示一个按钮
QGraphicsScene 本身不支持显示按钮,但是可以通过自定义 QGraphicsItem 实现一个按钮,并将其添加到 QGraphicsScene 中显示。
以下是一个简单的示例代码,用于创建一个 QGraphicsItem 按钮,并将其添加到 QGraphicsScene 中显示:
```python
from PyQt5.QtWidgets import QGraphicsItem, QGraphicsScene, QGraphicsTextItem, QGraphicsRectItem, QApplication, QGraphicsView, QGraphicsProxyWidget, QPushButton, QWidget
from PyQt5.QtCore import QRectF
from PyQt5.QtGui import QColor
class Button(QGraphicsItem):
def __init__(self, text, parent=None):
super(Button, self).__init__(parent)
self.text = QGraphicsTextItem(text, self)
self.text.setDefaultTextColor(QColor(255, 255, 255))
rect = self.text.boundingRect()
self.rect = QGraphicsRectItem(rect, self)
self.rect.setBrush(QColor(0, 0, 255))
def boundingRect(self):
return QRectF(self.rect.rect())
def paint(self, painter, option, widget=None):
pass
app = QApplication([])
scene = QGraphicsScene()
button = QPushButton('Button')
widget = QWidget()
layout = QVBoxLayout()
layout.addWidget(button)
widget.setLayout(layout)
proxy = QGraphicsProxyWidget()
proxy.setWidget(widget)
scene.addItem(proxy)
buttonItem = Button('Button Item')
buttonItem.setPos(100, 100)
scene.addItem(buttonItem)
view = QGraphicsView(scene)
view.show()
app.exec_()
```
这个示例代码中,我们创建了一个名为 Button 的自定义 QGraphicsItem 类,它包含一个 QGraphicsTextItem 和一个 QGraphicsRectItem,用于显示按钮的文本和背景色。然后,我们将一个 QPushButton 添加到一个 QWidget 中,并将 QWidget 包装成 QGraphicsProxyWidget,然后将 QGraphicsProxyWidget 添加到 QGraphicsScene 中,实现了一个按钮的显示。最后,我们创建了一个 ButtonItem 实例,并将其添加到 QGraphicsScene 中,以显示自定义按钮。
阅读全文
相关推荐
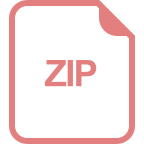
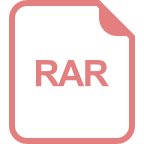
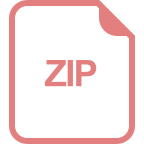
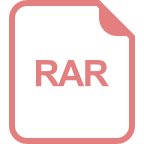
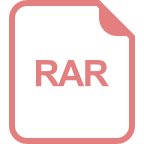
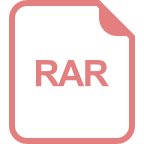
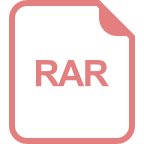
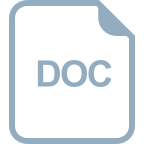








