使用C++语言,利用单链表实现职工信息的综合运算
时间: 2024-09-28 11:11:45 浏览: 66
在C++中,我们可以使用单链表来存储职工的信息,并实现一些基本的综合运算,如添加、删除和查找员工等。首先,我们需要定义一个节点结构体,包含职工的姓名、职位等属性,以及指向下一个节点的指针:
```cpp
struct Employee {
std::string name;
std::string position;
struct Employee* next;
};
```
然后,可以创建相应的链表操作函数:
1. **添加员工**:
```cpp
void addEmployee(Employee*& head, const Employee& newEmp) {
Employee* newNode = new Employee();
newNode->name = newEmp.name;
newNode->position = newEmp.position;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
} else {
Employee* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
```
2. **删除员工**:
```cpp
void deleteEmployee(Employee*& head, const std::string& name) {
if (head == nullptr) return;
if (head->name == name) {
Employee* temp = head;
head = head->next;
delete temp;
return;
}
Employee* current = head;
while (current->next != nullptr && current->next->name != name) {
current = current->next;
}
if (current->next != nullptr) {
Employee* temp = current->next;
current->next = current->next->next;
delete temp;
}
}
```
3. **查找员工**:
```cpp
Employee* findEmployee(const Employee* head, const std::string& name) {
Employee* current = head;
while (current != nullptr) {
if (current->name == name) return current;
current = current->next;
}
return nullptr; // 如果未找到,返回nullptr
}
```
这些基础操作结合在一起,就可以对职工信息进行综合处理,比如查找某个职位的所有员工,或者按照姓名排序等。
阅读全文
相关推荐



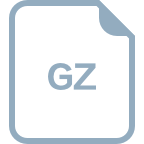

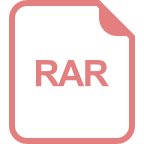
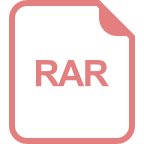






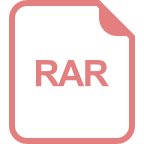


