DistributedDataParallel(module=model, device_ids=device_ids, **self._ddp_kwargs)
时间: 2024-04-14 07:32:03 浏览: 139
`DistributedDataParallel`(简称DDP)是PyTorch中的一个模型并行化工具,用于在分布式环境中对模型进行并行训练。给定一个模型和设备ID列表,以及其他可选的参数,`DistributedDataParallel`会在指定的设备上创建多个副本,并在每个副本上运行模型的不同部分,从而加速训练过程。
在给出的代码中,`DistributedDataParallel`的构造函数被调用,它接受以下参数:
- `module`: 要并行化的模型。通常是PyTorch的`nn.Module`子类的实例。
- `device_ids`: 设备ID列表,指定要在哪些设备上进行模型并行化。可以是单个设备ID或设备ID的列表。
- `**self._ddp_kwargs`: 其他可选参数。这里使用了`**`语法表示将字典中的键值对以关键字参数的形式传递给构造函数。
`DistributedDataParallel`会自动将模型的参数分发到指定的设备上,并将输入数据划分为小批量进行并行计算。它还提供了同步机制,用于在多个设备之间进行梯度聚合和参数更新。
请注意,使用`DistributedDataParallel`需要在分布式训练环境中运行代码,并设置适当的分布式配置。这通常涉及使用`torch.distributed`包中的函数来初始化和设置分布式训练环境。
阅读全文
相关推荐
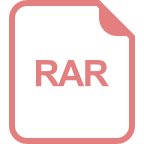
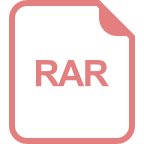
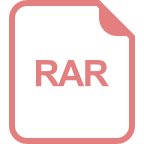
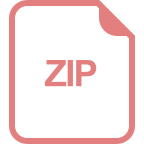
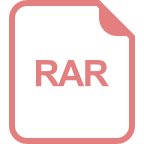
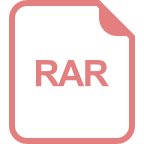
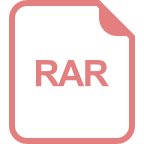
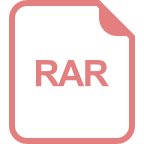
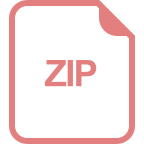
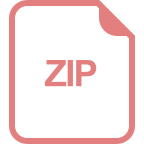
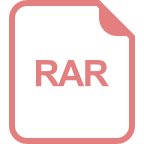
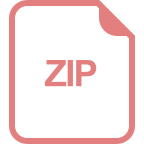
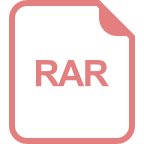
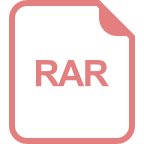
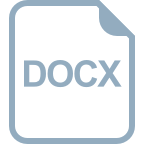