输入一个实数,统计其整数的位数。C语言
时间: 2024-09-20 09:01:56 浏览: 23
在C语言中,统计一个实数(double类型)的整数部分的位数并不是一个直接的操作,因为实数不是固定位数的,它们可以是无限精度的。不过,如果你有一个浮点数的整数值,你可以通过将它转换为整型,然后计算整型的位数来得到大致的整数位数。下面是一个简单的示例:
```c
#include <stdio.h>
#include <math.h>
// 计算整数部分位数的辅助函数
int count_digits(int n) {
if (n == 0)
return 1;
return log10(n) + 1; // 使用log10函数找到最小的10的幂次大于n
}
int main() {
double num;
printf("请输入一个实数:");
scanf("%lf", &num);
// 取整数部分并转换为整型
int integer = (int)(num * pow(10, ceil(log10(fabs(num)))));
// 统计整数位数
int digit_count = count_digits(integer);
printf("整数部分有 %d 位。\n", digit_count);
return 0;
}
```
这个程序首先获取输入的实数,将其四舍五入到最接近的整数,然后计算该整数的位数。但是请注意,这只是一个近似值,对于非常大的负数或者非十进制小数,结果可能会不准确。
相关问题
用C语言编写代码,输入一个实数,求整数部分位数
我可以提供一个简单的示例代码:int count = 0; double number; printf("请输入一个实数:"); scanf("%lf", &number); int int_part = (int)number; while(int_part > 0) { count++; int_part /= 10; } printf("该实数的整数部分位数为%d位。", count);
c语言 从键盘输入两个正实数,位数不超过200,试判断这两个实数是否完全相等。注意输入的实数整数部分可能有前导0,小数部分可能有末尾0。
在C语言中,判断两个实数是否完全相等,我们需要先读取用户的输入,并将其转换为浮点数。由于涉及到字符串处理和浮点数比较,可以按照以下步骤操作:
1. 使用`scanf`函数从键盘读入两个字符串,分别表示两个实数,格式化字符串可以指定最多读取200个字符,例如:`char str1[201], str2[201];`
2. 确保输入的是有效的数字,需要检查每个字符串是否只包含数字(包括'.'作为小数点)以及可能的前导和尾随的零。可以遍历字符串,如果遇到非数字字符则跳出循环。
3. 将字符串转换为浮点数。C语言本身没有直接将字符串转为浮点的函数,但可以借助`strtol`函数获取整数部分,然后加上小数点后的部分。需要注意处理小数点的位置和精度。
4. 比较两个浮点数是否相等。由于浮点数可能会有微小的精度误差,通常我们使用`fabs(a - b) < ε`这样的条件来判断,其中`a`和`b`是待比较的浮点数,`ε`是一个非常小的正数。
下面是一个简单的示例代码片段:
```c
#include <stdio.h>
#include <string.h>
#include <math.h>
int is_number(char *s) {
while (*s && *s >= '0' && *s <= '9') s++;
return (*s == '.') || !*s;
}
double atof_custom(char *str) {
int pos = 0, neg = 0;
if (*str == '-') {
neg = 1;
str++;
}
while (is_number(str)) {
pos *= 10;
pos += (str[0] - '0');
str++;
}
if (*str == '.') {
str++;
double frac = 0.1;
while (is_number(str)) {
frac *= 10;
frac += (str[0] - '0');
str++;
}
return neg ? -frac + pos : pos + frac;
} else {
return neg ? -pos : pos;
}
}
int main() {
char str1[201], str2[201];
printf("请输入第一个实数(最多200位):");
fgets(str1, sizeof(str1), stdin);
str1[strcspn(str1, "\n")] = '\0'; // 移除换行符
printf("请输入第二个实数(最多200位):");
fgets(str2, sizeof(str2), stdin);
str2[strcspn(str2, "\n")] = '\0';
double a = atof_custom(str1);
double b = atof_custom(str2);
if (fabs(a - b) < DBL_EPSILON) {
printf("两个实数完全相等。\n");
} else {
printf("两个实数不完全相等。\n");
}
return 0;
}
```
相关推荐
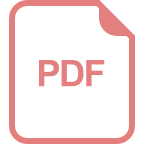
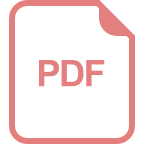
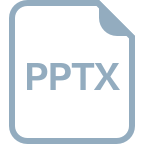
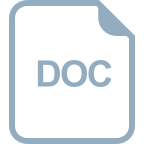
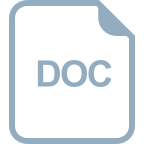
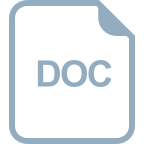
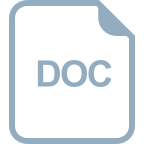








