python如何实现在划分好的训练集上训练模型,根据模型在验证集上的效果调整超参数
时间: 2024-11-20 17:37:05 浏览: 3
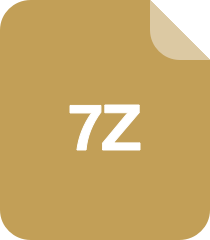
图像分类数据集:8种蔬菜识别【包括划分好的数据、类别字典文件、python数据可视化脚本】
在Python中,特别是在深度学习中,我们通常使用如Scikit-Learn、Keras或PyTorch等库来实现这种训练-验证流程,其中会涉及到交叉验证(Cross-validation)来评估模型性能并调整超参数。以下是基本步骤:
1. **数据分割**:首先将数据划分为训练集、验证集和测试集。一般比例可能是70%的数据用于训练,15%用于验证,剩下15%用于最终测试。可以使用`train_test_split`函数(来自sklearn.model_selection模块)来做到这一点。
```python
from sklearn.model_selection import train_test_split
X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.2, random_state=42)
```
2. **模型训练**:使用训练数据训练模型。
```python
model = MyModel() # 假设MyModel是你定义的模型
model.fit(X_train, y_train)
```
3. **交叉验证**:为了更准确地评估模型性能,我们会用到交叉验证。比如K折交叉验证(K-Fold CV),对数据进行多次分割,每次用一部分数据做验证,其余做训练。
```python
from sklearn.model_selection import cross_val_score
scores = cross_val_score(model, X_train, y_train, cv=5) # 5-fold CV
mean_score = scores.mean()
std_dev = scores.std()
```
4. **超参数调整**:使用如GridSearchCV或RandomizedSearchCV(同样来自sklearn.model_selection)来搜索最优超参数组合。这里超参数调整是在验证集上进行的,因为我们要找到的是能在新数据上表现良好的参数。
```python
from sklearn.model_selection import GridSearchCV
param_grid = {'learning_rate': [0.001, 0.01], 'batch_size': [32, 64]}
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X_train, y_train)
best_params = grid_search.best_params_
best_model = grid_search.best_estimator_
```
5. **评估和优化**:最后,在验证集上再次评估调整后的模型,并在必要时在测试集上做最终评估。
记得在整个过程中记录每个尝试的性能,以便了解哪些超参数组合最有效。
阅读全文
相关推荐
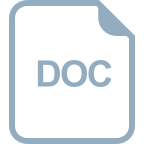
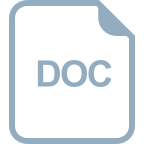





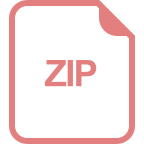
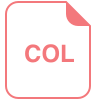
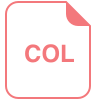







