c++字母小写转大写
时间: 2024-10-18 07:19:57 浏览: 35
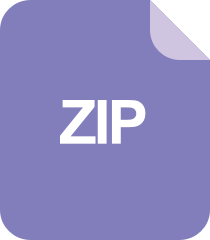
cpp代码-将小写字母变成大写字母,其余原样输出
在C++中,将字符串中的所有字母从小写转换为大写通常涉及到遍历字符串并使用库函数`toupper()`。`toupper()`是ctype.h头文件中的函数,它接受一个字符作为输入,并返回对应的大写字母。下面是一个简单的示例:
```cpp
#include <iostream>
#include <cctype> // 包含toupper()函数
std::string to_upper_case(std::string str) {
for (char& ch : str) { // 使用引用修改原始字符串中的字符
if (islower(ch)) { // 判断字符是否为小写
ch = toupper(ch); // 转换为大写
}
}
return str;
}
int main() {
std::string input = "hello world";
std::cout << "Original: " << input << "\n";
std::cout << "Uppercased: " << to_upper_case(input) << "\n";
return 0;
}
```
在这个例子中,`to_upper_case`函数接收一个字符串,通过迭代器逐个处理字符,如果遇到小写字母,则用`toupper()`将其转换为大写。
阅读全文
相关推荐
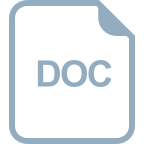
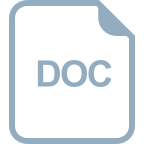












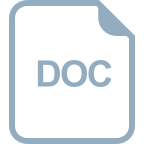
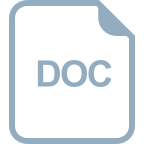
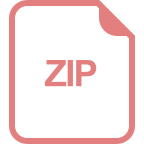