springboot上传oss文件流
时间: 2024-09-11 16:00:43 浏览: 16
Spring Boot 中上传文件到阿里云OSS (Object Storage Service) 的流程通常包括以下几个步骤:
1. 配置依赖:首先,在你的`pom.xml`中添加 Alibaba Cloud SDK 的依赖,比如 `spring-cloud-starter-alibaba-nacos-discovery` 和 `aliyun-sdk-core`。
```xml
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-oss</artifactId>
</dependency>
```
2. 实现上传服务:创建一个 Spring Bean,例如 `OssService`,用于处理上传操作。这个类通常会包含一个构造函数接收OSS客户端实例,并封装 OSS 文件上传的方法。
```java
import com.aliyuncs.IAcsClient;
import com.aliyuncs.OSSClientBuilder;
import com.aliyuncs.exceptions.ClientException;
import com.aliyuncs.exceptions.ServerException;
public class OssService {
private final IAcsClient ossClient;
public OssService(String endpoint, String accessKeyId, String accessKeySecret, String bucketName) {
this.ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
}
public void uploadFileToOSS(InputStream inputStream, String objectKey) throws ClientException, ServerException {
ossClient.putObject(bucketName, objectKey, inputStream);
ossClient.shutdown();
}
}
```
3. 调用上传方法:在需要上传文件的地方,你可以注入 `OssService` 并调用 `uploadFileToOSS` 方法,传入文件流和对象键(objectKey)。
```java
@Autowired
private OssService ossService;
public void uploadFileToOSS(File file, String fileName) {
try (InputStream inputStream = new FileInputStream(file)) {
ossService.uploadFileToOSS(inputStream, fileName);
} catch (IOException e) {
log.error("Failed to upload file", e);
}
}
```
相关推荐
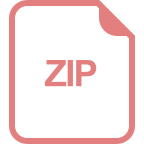
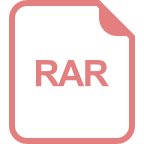
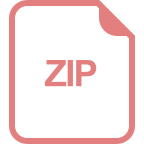














