C语言如何读取和写入二进制文件?
时间: 2024-09-19 18:11:28 浏览: 104
在C语言中,读取和写入二进制文件通常需要使用标准库中的文件操作函数,包括`fopen()`、`fwrite()`、`fclose()`等。以下是一个简单的例子:
**读取二进制文件:**
```c
#include <stdio.h>
int main() {
FILE* file = fopen("binary_file.bin", "rb"); // rb 表示二进制模式读取
if (file == NULL) {
printf("Failed to open the file.\n");
return 1;
}
char buffer[100]; // 假设你想读取100字节的数据
size_t bytes_read = fread(buffer, sizeof(char), 100, file);
if (bytes_read != 100) {
printf("Error reading data from the file.\n");
} else {
printf("Data read successfully: %s\n", buffer); // 输出读取的内容
}
fclose(file);
return 0;
}
```
**写入二进制文件:**
```c
#include <stdio.h>
int main() {
FILE* file = fopen("binary_file.bin", "wb"); // wb 表示二进制模式写入
if (file == NULL) {
printf("Failed to open the file.\n");
return 1;
}
char data[] = {0x01, 0x02, 0x03, ...}; // 你要写入的二进制数据
size_t bytes_written = fwrite(data, sizeof(char), sizeof(data), file);
if (bytes_written != sizeof(data)) {
printf("Error writing to the file.\n");
} else {
printf("Data written successfully.\n");
}
fclose(file);
return 0;
}
```
记住在完成文件操作后关闭文件以释放资源。
阅读全文
相关推荐
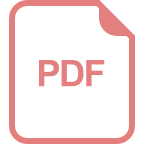
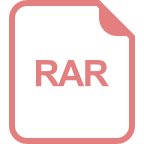
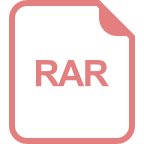

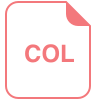


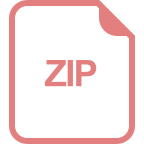
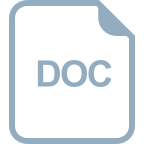




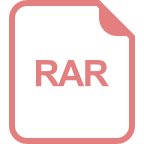
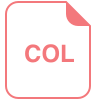
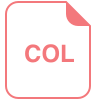


