arrary find
时间: 2024-12-25 13:16:05 浏览: 2
数组的`find()`方法通常是在JavaScript中用于查找符合条件的第一个元素。这个方法会遍历数组,直到找到第一个满足给定测试函数的元素,或者搜索完整个数组都没有找到匹配项。它的语法通常是这样的:
```javascript
array.find(callback(element[, index[, array]])[, thisArg])
```
- `callback`: 函数,它会被数组中的每个元素依次调用。如果该元素通过测试(即回调函数返回`true`),则`find()`将停止并返回该元素。
- `element`: 当前正在检查的数组元素值。
- `index` (可选): 元素的索引,在回调函数内部可用。
- `array` (可选): 调用`find`时所在的数组,这里默认为上下文中的数组。
- `thisArg` (可选): 在回调函数中使用的`this`引用。
例如:
```javascript
const numbers = [1, 2, 3, 4, 5];
const foundIndex = numbers.find(item => item > 3); // 返回4,因为4是第一个大于3的元素
```
如果所有元素都不满足条件,`find()`将返回`undefined`。
相关问题
es6 array find
ES6中的数组.find()方法用于找到数组中满足给定测试函数的第一个元素,并返回该元素。如果找不到符合条件的元素,则返回undefined。
.find()方法接受一个测试函数作为参数,该函数接受三个参数:当前正在遍历的元素,当前元素的索引和原始数组。测试函数应该返回一个布尔值,用于判断当前元素是否满足条件。
使用示例:
```javascript
const arr = [1, 2, 3, 4, 5];
// 找到第一个大于3的元素
const result = arr.find(element => element > 3);
console.log(result); // 4
// 找到第一个能被2整除的元素
const evenNumber = arr.find(element => element % 2 === 0);
console.log(evenNumber); // 2
// 找到第一个索引大于等于2的元素
const indexElement = arr.find((element, index) => index >= 2);
console.log(indexElement); // 3
// 没有找到符合条件的元素
const notFound = arr.find(element => element > 5);
console.log(notFound); // undefined
```
总结:ES6中的数组.find()方法可以用于查找满足条件的第一个元素。可以根据当前元素的值、索引或原始数组来编写测试函数。如果找到符合条件的元素,则返回该元素;否则返回undefined。
js array find
在JavaScript中,`Array.prototype.find()`是一个数组方法,用于返回数组中第一个满足指定条件的元素。它接受一个回调函数作为参数,该回调函数可以接受三个参数:当前循环的项、循环项的下标和当前循环的数组。在回调函数中,你可以使用条件语句或其他逻辑来判断当前项是否符合你的要求。如果找到了符合条件的元素,`find()`方法将立即返回该元素,并且不会继续循环数组。如果数组中没有符合条件的元素,则返回`undefined`。如果传入了第二个参数`thisValue`,在回调函数中的`this`关键字将指向`thisValue`。下面是几个示例:
示例1: 返回数组中第一个大于6的值
```javascript
var array = [1, 2, 3, 4, 5, 6, 6, 6];
var result = array.find(function(item, index, arr) {
return item > 6;
});
console.log(result); // 输出: undefined
```
示例2: 返回数组中第一个大于5的值,并输出数组的变化
```javascript
var array = [1, 2, 3, 4, 5, 6, 6, 6];
var result = array.find(function(item, index, arr) {
return item > 5;
});
console.log(result); // 输出: 6
console.log(array); // 输出: [1, 2, 3, 4, 5, 6, 6, 6]
```
示例3: 使用箭头函数简化代码,返回数组中第一个大于1的值
```javascript
let arr1 = [1, 2, 3, 4, 5];
let num = arr1.find(item => item > 1);
console.log(num); // 输出: 2
```
总结来说,`Array.prototype.find()`方法是用于在数组中查找符合条件的第一个元素的方法,它非常灵活,可以根据不同的需求编写不同的回调函数来实现各种功能。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [js数组find方法详解](https://blog.csdn.net/qrbd4558/article/details/115656863)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* [JS中find方法的使用](https://blog.csdn.net/m0_59168984/article/details/121557906)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
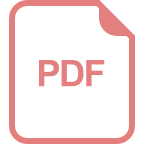
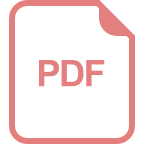
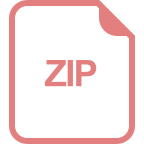
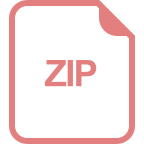
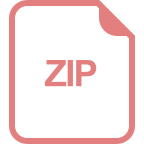
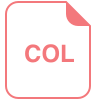


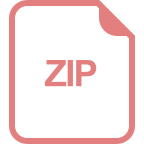
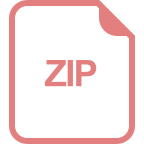
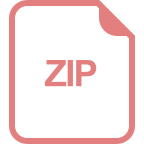
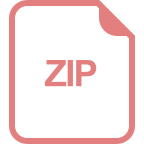
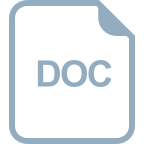



