df = df.dropna(subset=[col for col in df.columns if col != column_name]
时间: 2024-10-23 14:11:13 浏览: 12
这段Python代码是在使用Pandas库对DataFrame(df)进行操作,其目的是在一个DataFrame中排除(drop)特定列(column_name)及其对应的缺失值。这里的关键在于使用了列表推导式(list comprehension)来筛选出除了目标列(column_name)之外的所有列(col for col in df.columns if col != column_name)作为subset参数传递给dropna函数。
具体解释如下:
1. `df.columns`: 获取DataFrame df的所有列名。
2. `if col != column_name`: 遍历每一列名,如果列名不是你要排除的那个(column_name),则保留该列。
3. `[col for ...]:` 构造一个新的列表,包含符合条件的列名。
4. `df.dropna(subset=[...])`: 将这个新列表传入dropna函数,指定仅基于这个列表中的列检查缺失值,然后删除那些所有指定列都存在缺失值的行。
举个例子,如果你有一个DataFrame,其中包含'column_name'和其它几列,而你想删除除'column_name'外其他列中存在缺失值的所有行,你会这样做:
```python
# 假设 df 是一个 DataFrame,column_name 是你想排除的那一列名
df_no_missing = df.dropna(subset=[col for col in df.columns if col != column_name])
```
相关问题
优化代码 def cluster_format(self, start_time, end_time, save_on=True, data_clean=False, data_name=None): """ local format function is to format data from beihang. :param start_time: :param end_time: :return: """ # 户用簇级数据清洗 if data_clean: unused_index_col = [i for i in self.df.columns if 'Unnamed' in i] self.df.drop(columns=unused_index_col, inplace=True) self.df.drop_duplicates(inplace=True, ignore_index=True) self.df.reset_index(drop=True, inplace=True) dupli_header_lines = np.where(self.df['sendtime'] == 'sendtime')[0] self.df.drop(index=dupli_header_lines, inplace=True) self.df = self.df.apply(pd.to_numeric, errors='ignore') self.df['sendtime'] = pd.to_datetime(self.df['sendtime']) self.df.sort_values(by='sendtime', inplace=True, ignore_index=True) self.df.to_csv(data_name, index=False) # 调用基本格式化处理 self.df = super().format(start_time, end_time) module_number_register = np.unique(self.df['bat_module_num']) # if registered m_num is 0 and not changed, there is no module data if not np.any(module_number_register): logger.logger.warning("No module data!") sys.exit() if 'bat_module_voltage_00' in self.df.columns: volt_ref = 'bat_module_voltage_00' elif 'bat_module_voltage_01' in self.df.columns: volt_ref = 'bat_module_voltage_01' elif 'bat_module_voltage_02' in self.df.columns: volt_ref = 'bat_module_voltage_02' else: logger.logger.warning("No module data!") sys.exit() self.df.dropna(axis=0, subset=[volt_ref], inplace=True) self.df.reset_index(drop=True, inplace=True) self.headers = list(self.df.columns) # time duration of a cluster self.length = len(self.df) if self.length == 0: logger.logger.warning("After cluster data clean, no effective data!") raise ValueError("No effective data after cluster data clean.") self.cluster_stats(save_on) for m in range(self.mod_num): print(self.clusterid, self.mod_num) self.module_list.append(np.unique(self.df[f'bat_module_sn_{str(m).zfill(2)}'].dropna())[0])
Here are some possible optimizations for the given code:
1. Instead of using a list comprehension to find columns with 'Unnamed' in their names, you can use the `filter()` function along with a lambda function to achieve the same result in a more concise way:
```
unused_index_col = list(filter(lambda x: 'Unnamed' in x, self.df.columns))
```
2. Instead of dropping duplicates and resetting the index separately, you can use the `drop_duplicates()` function with the `ignore_index` parameter set to `True` to achieve both in one step:
```
self.df.drop_duplicates(inplace=True, ignore_index=True)
```
3. Instead of using `sys.exit()` to terminate the program when there is no module data, you can raise a `ValueError` with an appropriate error message:
```
raise ValueError("No module data!")
```
4. Instead of using a series of `if` statements to find the voltage reference column, you can use the `loc` accessor with a boolean mask to select the first column that starts with 'bat_module_voltage':
```
volt_ref_col = self.df.columns[self.df.columns.str.startswith('bat_module_voltage')][0]
```
5. Instead of using a loop to append a single item to a list, you can use the `append()` method directly:
```
self.module_list.append(np.unique(self.df[f'bat_module_sn_{str(m).zfill(2)}'].dropna())[0])
```
By applying these optimizations, the code can become more concise and efficient.
给一个例子,他现在是一列,还是列表,还是df结构
这段代码创建的是一个包含多个列的列表,每个元素都是一个 PySpark 的 `Column` 对象,用于表示 DataFrame 中的每个列。这个列表中的每个元素都是由 `self.config.deduplication_key_columns` 属性中提供的列名构建而成。
例如,如果 `self.config.deduplication_key_columns` 属性被设置为 `['name', 'age', 'gender']`,那么这段代码创建的 `key_columns` 列表将包含三个元素,每个元素都是一个 `Column` 对象,用于表示 DataFrame 中的 'name'、'age' 和 'gender' 三个列。
在这个例子中,`key_columns` 列表可以被用于对 DataFrame 进行去重操作,例如:
```python
from pyspark.sql.functions import *
df = spark.createDataFrame([(1, "John", 25, "M"), (2, "Mary", 30, "F"), (3, "John", 25, "M"), (4, "Mary", 28, "F")], ["id", "name", "age", "gender"])
key_columns = [col(column_name) for column_name in ['name', 'age', 'gender']]
deduplicated_df = df.dropDuplicates(subset=key_columns)
deduplicated_df.show()
```
这个例子中,我们创建了一个 DataFrame `df`,包含四列 'id'、'name'、'age' 和 'gender'。然后,我们使用 `key_columns` 列表对 DataFrame 进行去重操作,只保留 'name'、'age' 和 'gender' 三列的数值完全相同的行。最后,我们使用 `show()` 函数展示去重后的 DataFrame。
阅读全文
相关推荐
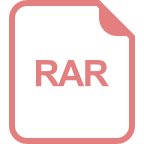
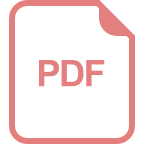
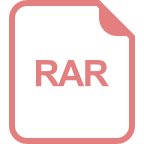


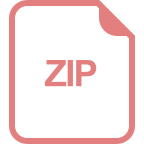
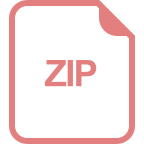
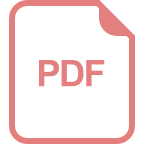
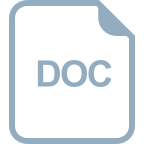
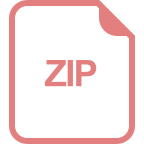
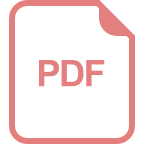
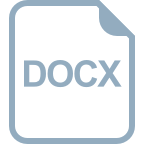