c#sortedlist排序
时间: 2024-09-18 22:11:15 浏览: 10
在C#中,`SortedList`用于存储键值对并按照指定的排序方式进行操作。其默认行为是根据键的自然顺序进行排序,但可以自定义排序规则。以下是基本用法:
1. **基本用法**[^1]:
```csharp
// 创建一个SortedDictionary实例,键是字符串,值是整数
SortedList<string, int> dict = new SortedList<string, int>();
// 添加键值对
dict.Add("Apple", 1);
dict.Add("Banana", 2);
// 获取排序后的键值对
foreach (KeyValuePair<string, int> pair in dict)
{
Console.WriteLine($"{pair.Key}: {pair.Value}");
}
```
2. **自定义排序规则**:
如果要按照其他条件排序,可以创建一个实现了`IComparer<T>`接口的比较器类,然后传递给构造函数或设置`IComparer<TKey>`属性。
```csharp
class MyCustomComparer : IComparer<string>
{
public int Compare(string x, string y)
{
return string.Compare(x, y, StringComparison.OrdinalIgnoreCase);
}
}
var comparer = new MyCustomComparer();
SortedList<string, int> dictWithCustomSort = new SortedList<string, int>(comparer);
// 使用自定义比较器添加和访问元素
dictWithCustomSort.Add("Zebra", 3);
dictWithCustomSort.Add("Dog", 1);
```
3. **注意事项**:
- `SortedList`是线程不安全的,如果在多线程环境下使用,需要自己同步以保证数据一致性。
- 对于大型数据集,`SortedList`可能会比其他更高效的排序算法如`Dictionary<TKey, TValue>`稍慢,因为它需要维护键的排序。
相关推荐
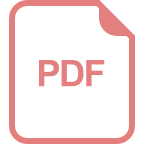
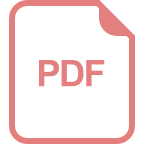
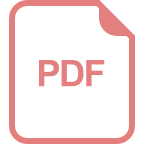














