字符串中的单词反转,同时保留空格和初始单词顺序,用C语言
时间: 2024-09-12 18:15:33 浏览: 48
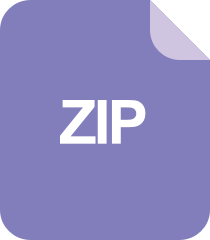
c语言面试题之双指针反转字符串中的单词III.zip
在C语言中,可以将字符串分割成单词,然后对每个单词单独反转,最后再连接回原字符串。这里有一个简单的实现方法,它使用了`strtok()`函数来处理单词,并通过`sprintf()`函数构建新的反转字符串:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
// 反转单个单词并保持空格不变
void reverseWord(char *word) {
int start = 0, end = 0;
while (isspace(*word)) word++; // 跳过开头的空格
end = word;
while (*end && !isspace(*end)) end++;
// 反转单词
for (int i = start; i < end; ++i) {
char temp = word[i];
word[i] = word[end - 1 - i];
word[end - 1 - i] = temp;
}
}
// 将字符串反转单词,保留空格和原始顺序
char* reverseWordsInString(char *str) {
char reversed[1024]; // 假设输入字符串不超过1024个字符
char *token = strtok(str, " "); // 分割词
while (token) {
reverseWord(token); // 反转当前词
strcat(reversed, token); // 添加到结果
strcat(reversed, " "); // 添加空格
token = strtok(NULL, " "); // 获取下一个词
}
reversed[strlen(reversed) - 1] = '\0'; // 删除多余的空格
return reversed;
}
int main() {
char str[] = "Hello World, This is a test.";
char *reversedStr = reverseWordsInString(str);
printf("Reversed words with spaces: %s\n", reversedStr);
free(reversedStr); // 释放内存
return 0;
}
```
阅读全文
相关推荐
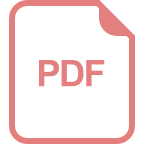
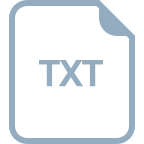





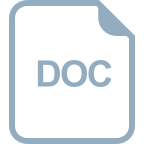
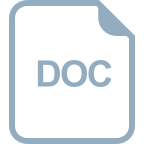




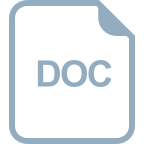
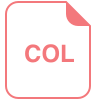
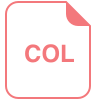
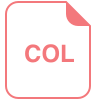
