python持续写入文件
时间: 2023-11-08 09:02:39 浏览: 57
在Python中,你可以使用文件的打开模式参数来实现持续写入文件。使用 "a" 模式进行追加写入,可以在文件末尾添加新的内容,而不会覆盖原有的内容。下面是一个示例代码:
```python
# 打开文件以追加写入的方式
file = open("example.txt", "a")
# 写入内容
file.write("这是新的一行\n")
# 关闭文件
file.close()
```
这段代码将在 "example.txt" 文件中追加写入一行新的内容。你可以多次调用 `file.write()` 函数来持续写入多行内容。
如果你希望每次写入内容都另起一行,你可以在写入的文本末尾添加换行符 `\n`。
相关问题
python从键盘输入一些字符串,并写入磁盘文件,直到输入¥为止,需要全部代码
好的,我知道了。下面是这个要求的 Python 代码:
```python
# 打开文件
with open('output.txt', 'w') as file:
while True:
# 从键盘读取输入
s = input()
# 如果输入为 $ 就退出循环
if s == '¥':
break
# 将输入写入文件
file.write(s + '\n')
```
这个程序会打开一个名为 `output.txt` 的文件,并在每次从键盘输入一行字符串后将其写入文件。输入的过程会一直持续,直到输入了一个 `¥` 符号,此时程序会结束并关闭文件。请注意,文件会被写入到当前工作目录下。
python如何实现边循环爬取json边写入excel
要实现边循环爬取json边写入Excel,可以按照以下步骤进行操作:
1. 导入所需的库:
```python
import requests
import json
import openpyxl
```
2. 循环请求API并获取JSON数据:
```python
url = 'https://example.com/api/data'
response = requests.get(url)
data = json.loads(response.text)
```
3. 创建一个Excel文件并设置活动工作表:
```python
workbook = openpyxl.Workbook()
worksheet = workbook.active
```
4. 循环遍历JSON数据并将其写入Excel行:
```python
for row in data:
worksheet.append(row.values())
```
5. 保存Excel文件:
```python
workbook.save('data.xlsx')
```
6. 将以上步骤放入一个循环中,以便可以持续爬取JSON数据并将其写入Excel中:
```python
while True:
response = requests.get(url)
data = json.loads(response.text)
workbook = openpyxl.Workbook()
worksheet = workbook.active
for row in data:
worksheet.append(row.values())
workbook.save('data.xlsx')
```
这样就可以实现边循环爬取JSON边写入Excel的操作了。
相关推荐
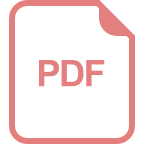
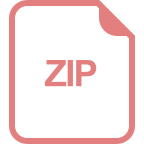
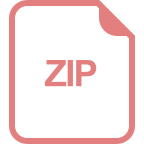












