Casting 'findViewById(...)' to 'TextView' is redundant
时间: 2024-12-14 18:15:08 浏览: 7
" Casting 'findViewById(...)' to 'TextView' is redundant" 这句话意味着当你在Android应用中通过 `findViewById()` 方法获取到视图控件后,将其强制转换成 `TextView` 类型是多余的。`findViewById()` 返回的是一个 `View` 对象,这是所有UI组件的基础类型。如果已经确认返回的结果确实是 `TextView`,那么直接使用它就不会有类型转换的问题,因为 `TextView` 是 `View` 的子类。
例如:
```java
View view = findViewById(R.id.my_text_view);
if (view instanceof TextView) {
TextView textView = (TextView) view;
// 现在可以直接操作textView了
String text = textView.getText().toString();
}
```
在这种情况下,如果`view`确实是个`TextView`,强制转换是不必要的,因为它已经是正确的类型。然而,如果存在不确定性,即`view`可能是其他类型的`View`,这种转换就很有用了,以避免隐式转换可能导致的潜在问题。
相关问题
com.alibaba.fastjson.JSONObject cannot be cast to
java.lang.String.
This error occurs when you try to cast an object of type `com.alibaba.fastjson.JSONObject` to a `String` type. In Java, casting is only allowed between compatible types, and a `JSONObject` cannot be directly cast to a `String`.
To resolve this issue, you need to extract the desired value from the `JSONObject` and convert it to a `String`. You can use the `toString()` method provided by the `JSONObject` class to convert the entire object to a `String`. If you want a specific value from the JSON object, you can retrieve it using the appropriate methods provided by the `JSONObject` class and then convert it to a `String`.
Here's an example of how you can extract a value from a `JSONObject` and convert it to a `String`:
```java
com.alibaba.fastjson.JSONObject jsonObject = ...; // your JSONObject
// Get the desired value from the JSONObject
Object value = jsonObject.get("key");
// Convert the value to String
String stringValue = String.valueOf(value);
```
Make sure to replace `"key"` with the actual key of the value you want to extract from the JSON object.
TypeError: Failed to convert object of type <class 'list'> to Tensor. Contents: [None, None]. Consider casting elements to a supported type.
这个错误通常出现在使用TensorFlow时,你把一个列表传递给了一个需要张量(tensor)类型的函数,但是列表并不是一个支持的类型。
通常情况下,将列表转换为张量类型可以使用以下代码:
```
import tensorflow as tf
lst = [1, 2, 3]
tensor = tf.convert_to_tensor(lst, dtype=tf.float32)
```
在这个例子中,我们使用 `tf.convert_to_tensor` 函数将列表 `lst` 转换为张量类型,并指定了张量的数据类型为 `tf.float32`。
如果你的列表 `lst` 包含 `None` 值,你需要在转换张量之前处理这些值。例如,你可以将所有的 `None` 替换为特定的值,如 `-1`。
```
lst = [1, None, 3]
lst = [-1 if x is None else x for x in lst]
tensor = tf.convert_to_tensor(lst, dtype=tf.float32)
```
这个例子中,我们使用列表推导式将所有的 `None` 替换为 `-1`,然后将修改后的列表转换为张量类型。
阅读全文
相关推荐
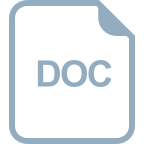
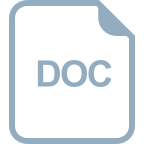
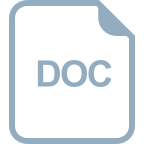
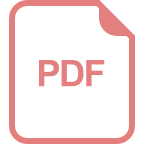
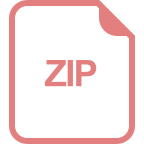
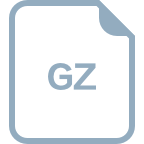
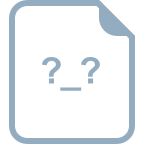
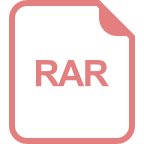
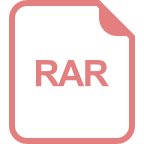
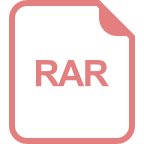
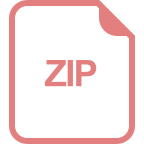




