哈希表实现功能的代码的c语言
时间: 2024-10-27 14:03:50 浏览: 31
哈希表(Hash Table)是一种常见的数据结构,它通过哈希函数将键(Key)映射到一个固定的位置,实现快速查找、插入和删除操作。在C语言中,我们可以使用数组和链表结合的方式来实现一个简单的哈希表。
下面是一个基本的哈希表实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 假设我们使用7作为哈希表的大小
#define TABLE_SIZE 7
// 哈希表的节点结构体
typedef struct {
char* key;
int value;
struct node* next; // 链接表用于解决冲突
} HashNode;
// 哈希表
typedef struct {
HashNode** table;
} HashTable;
// 哈希函数,这里简单地取模运算
int hash_function(char* key) {
return strlen(key) % TABLE_SIZE;
}
// 创建一个新的哈希表
HashTable* create_hash_table() {
HashTable* ht = (HashTable*)malloc(sizeof(HashTable));
ht->table = (HashNode**)calloc(TABLE_SIZE, sizeof(HashNode*));
for (int i = 0; i < TABLE_SIZE; i++) {
ht->table[i] = NULL;
}
return ht;
}
// 插入键值对
void insert(HashTable* ht, char* key, int value) {
int index = hash_function(key);
HashNode* newNode = (HashNode*)malloc(sizeof(HashNode));
newNode->key = strdup(key);
newNode->value = value;
newNode->next = ht->table[index];
ht->table[index] = newNode;
}
// 查找键对应的值
int find(HashTable* ht, char* key) {
int index = hash_function(key);
HashNode* current = ht->table[index];
while (current != NULL) {
if (strcmp(current->key, key) == 0) {
return current->value;
}
current = current->next;
}
return -1; // 如果找不到,返回-1表示未找到
}
// 删除键对应的值
void delete(HashTable* ht, char* key) {
int index = hash_function(key);
HashNode* current = ht->table[index];
HashNode* prev = NULL;
while (current != NULL) {
if (strcmp(current->key, key) == 0) {
if (prev == NULL) {
ht->table[index] = current->next;
} else {
prev->next = current->next;
}
free(current->key);
free(current);
return;
}
prev = current;
current = current->next;
}
}
// 示例用法
int main() {
HashTable* ht = create_hash_table();
insert(ht, "apple", 10);
insert(ht, "banana", 20);
printf("Find apple: %d\n", find(ht, "apple")); // 输出 10
delete(ht, "banana");
printf("Find banana after deletion: %d\n", find(ht, "banana")); // 输出 -1 (未找到)
return 0;
}
```
阅读全文
相关推荐





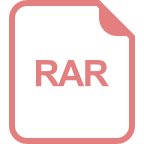



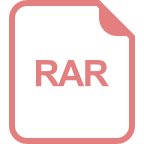








