哈希表设计C语言代码实现
时间: 2023-08-14 08:40:53 浏览: 82
以下是一个简单的哈希表设计 C 语言代码实现,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 100
typedef struct {
char* key;
int value;
} HashNode;
typedef struct {
HashNode** nodes;
int size;
} HashTable;
HashTable* createHashTable(int size) {
HashTable* hashtable = (HashTable*)malloc(sizeof(HashTable));
hashtable->nodes = (HashNode**)calloc(size, sizeof(HashNode*));
hashtable->size = size;
return hashtable;
}
int hash(char* key, int size) {
int hash = 0;
int len = strlen(key);
for (int i = 0; i < len; i++) {
hash = (hash * 31 + key[i]) % size;
}
return hash;
}
void put(HashTable* hashtable, char* key, int value) {
int hashValue = hash(key, hashtable->size);
HashNode* node = hashtable->nodes[hashValue];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
node->value = value;
return;
}
node = node->next;
}
node = (HashNode*)malloc(sizeof(HashNode));
node->key = key;
node->value = value;
node->next = hashtable->nodes[hashValue];
hashtable->nodes[hashValue] = node;
}
int get(HashTable* hashtable, char* key) {
int hashValue = hash(key, hashtable->size);
HashNode* node = hashtable->nodes[hashValue];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
return node->value;
}
node = node->next;
}
printf("Error: Key not found\n");
return -1;
}
void delete(HashTable* hashtable, char* key) {
int hashValue = hash(key, hashtable->size);
HashNode* node = hashtable->nodes[hashValue];
HashNode* prev = NULL;
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
if (prev == NULL) {
hashtable->nodes[hashValue] = node->next;
} else {
prev->next = node->next;
}
free(node);
return;
}
prev = node;
node = node->next;
}
printf("Error: Key not found\n");
}
void printHashTable(HashTable* hashtable) {
for (int i = 0; i < hashtable->size; i++) {
printf("%d: ", i);
HashNode* node = hashtable->nodes[i];
while (node != NULL) {
printf("(%s, %d) ", node->key, node->value);
node = node->next;
}
printf("\n");
}
}
int main() {
HashTable* hashtable = createHashTable(SIZE);
put(hashtable, "apple", 3);
put(hashtable, "banana", 2);
put(hashtable, "orange", 1);
printHashTable(hashtable);
printf("Get value of apple: %d\n", get(hashtable, "apple"));
delete(hashtable, "banana");
printHashTable(hashtable);
return 0;
}
```
解释一下上述代码:
- `HashTable` 结构体包含一个 `nodes` 数组,用来存储哈希表中的节点,以及 `size` 表示哈希表的大小。
- `HashNode` 结构体包含一个 `key` 字符串和一个 `value` 整数,表示键值对。
- `createHashTable` 函数创建一个哈希表,并返回其指针。
- `hash` 函数接收一个键和哈希表的大小,计算该键的哈希值,并返回对应哈希表中的位置。
- `put` 函数接收键值对,将其插入哈希表中。如果该键已经存在,则更新对应的值。
- `get` 函数接收一个键,返回对应的值。如果该键不存在,则输出错误信息,并返回 -1。
- `delete` 函数接收一个键,将其从哈希表中删除。如果该键不存在,则输出错误信息。
- `printHashTable` 函数打印哈希表的内容,用于调试。
- `main` 函数创建一个哈希表,插入若干键值对,然后调用各种函数测试其功能。
相关推荐
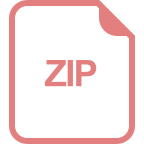
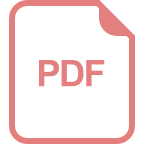















