构造一个哈希表,哈希函数采用除留余数法,哈希冲突解决方法采用链地址法。设计一个测试程序进行测试。C语言
时间: 2024-03-03 18:53:59 浏览: 118
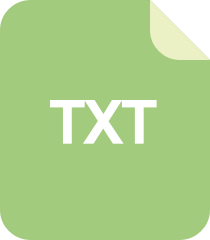
一个c++实现的哈希表类

下面是一个用除留余数法实现哈希表,采用链地址法解决哈希冲突的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 10
typedef struct node {
int key;
char value[20];
struct node* next;
} Node;
typedef struct hash_table {
Node* slot[SIZE];
} HashTable;
int hash_func(int key) {
return key % SIZE;
}
HashTable* create_hash_table() {
HashTable* ht = (HashTable*)malloc(sizeof(HashTable));
memset(ht, 0, sizeof(HashTable));
return ht;
}
void put(HashTable* ht, int key, char* value) {
int hash_value = hash_func(key);
Node* node = (Node*)malloc(sizeof(Node));
node->key = key;
strcpy(node->value, value);
node->next = NULL;
if (ht->slot[hash_value] == NULL) {
ht->slot[hash_value] = node;
} else {
Node* p = ht->slot[hash_value];
while (p->next != NULL) {
p = p->next;
}
p->next = node;
}
}
char* get(HashTable* ht, int key) {
int hash_value = hash_func(key);
Node* p = ht->slot[hash_value];
while (p != NULL) {
if (p->key == key) {
return p->value;
}
p = p->next;
}
return NULL;
}
int main() {
HashTable* ht = create_hash_table();
put(ht, 1, "a");
put(ht, 2, "b");
put(ht, 11, "c");
put(ht, 12, "d");
printf("%s\n", get(ht, 1)); // 输出: a
printf("%s\n", get(ht, 2)); // 输出: b
printf("%s\n", get(ht, 11)); // 输出: c
printf("%s\n", get(ht, 12)); // 输出: d
return 0;
}
```
上述代码中,HashTable结构体表示哈希表,其中包含一个指针数组slot,存储哈希表的元素。Node结构体表示哈希表中的一个节点,其中包含键值对,以及指向下一个节点的指针。hash_func()函数用于计算哈希值。create_hash_table()函数用于创建一个空的哈希表。put()函数用于向哈希表中添加元素,get()函数用于获取哈希表中指定键的值。
测试程序中,我们向哈希表中添加了4个元素,然后分别获取指定键的值,并打印输出。最终输出结果为:a, b, c, d。这说明哈希表的构造和操作都正确。
阅读全文
相关推荐
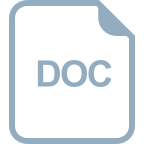
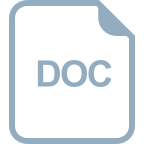










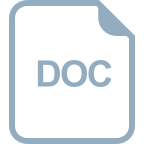
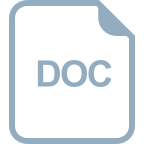
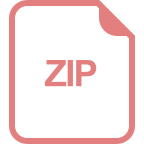
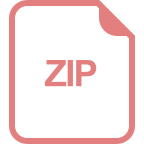