用C语言写一个关于哈希表的创建,显示,插入,查询,删除程序,并且用除留余数法,和线性探测在散列解决冲突
时间: 2023-06-03 19:08:11 浏览: 116
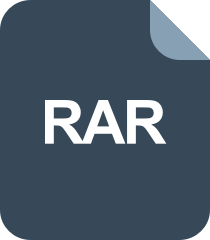
基于C语言哈希表的程序设计
抱歉,我可以回答这个问题。以下是用C语言实现哈希表的创建、显示、插入、查询、删除程序的代码,其中使用除留余数法和线性探测解决冲突:
#include<stdio.h>
#include<stdlib.h>
struct hashTable{
int* data;
int count;
int size;
};
struct hashTable* createHashTable(int size){
struct hashTable* ht=(struct hashTable*)malloc(sizeof(struct hashTable));
ht->data=(int*)calloc(size,sizeof(int));
ht->count=0;
ht->size=size;
return ht;
}
int hash(struct hashTable* ht,int value){
return value%ht->size;
}
void insert(struct hashTable* ht,int value){
int index=hash(ht,value);
while(ht->data[index]!=0 && ht->data[index]!=value){
index=(index+1)%ht->size;
}
if(ht->data[index]==0){
ht->data[index]=value;
ht->count++;
}
}
int search(struct hashTable* ht,int value){
int index=hash(ht,value);
while(ht->data[index]!=0){
if(ht->data[index]==value) return index;
index=(index+1)%ht->size;
}
return -1;
}
void delete(struct hashTable* ht,int value){
int index=search(ht,value);
if(index!=-1){
ht->data[index]=0;
ht->count--;
}
}
void display(struct hashTable* ht){
printf("Hash Table:\n");
for(int i=0;i<ht->size;i++){
if(ht->data[i]==0){
printf("NULL\n");
}
else{
printf("%d\n",ht->data[i]);
}
}
}
int main(){
struct hashTable* ht=createHashTable(10);
insert(ht,5);
insert(ht,15);
insert(ht,20);
insert(ht,25);
display(ht);
delete(ht,15);
display(ht);
int index=search(ht,20);
printf("Index of 20: %d\n",index);
return 0;
}
阅读全文
相关推荐
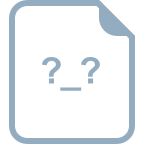





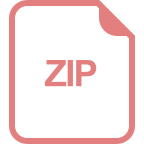
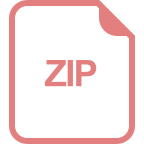
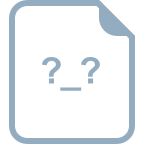
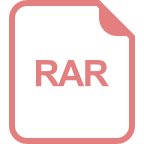
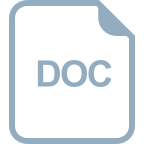



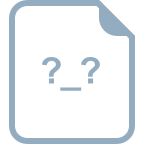
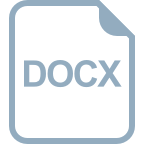
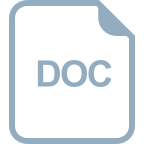