python 爬虫 定义一个 协程类 数据解析
时间: 2024-09-16 09:06:21 浏览: 113
在Python中,爬虫通常使用异步IO库如`asyncio`来创建协程,因为网络请求往往是耗时的操作,通过协程可以并发地处理多个请求,提高效率。首先,你需要定义一个基础的协程类,例如:
```python
import asyncio
from aiohttp import ClientSession
class CoroutineCrawler:
def __init__(self):
self.session = ClientSession()
async def fetch_page(self, url):
"""
使用asyncio和aiohttp发起GET请求
"""
async with self.session.get(url) as response:
data = await response.text()
return data
async def parse_data(self, data):
"""
解析数据的方法,可以根据实际需要定制
"""
# 这里可以使用BeautifulSoup、lxml等库解析HTML
parser_result = self.parse_html(data)
return parser_result
@staticmethod
def parse_html(html):
"""
示例中的静态解析函数
"""
# ... 实现数据提取逻辑
pass
async def run(self, urls):
tasks = [self.fetch_page(url) for url in urls]
responses = await asyncio.gather(*tasks)
parsed_data = [self.parse_data(response) for response in responses]
# 打印或进一步处理解析结果
for data in parsed_data:
print(data)
# 使用示例
crawler = CoroutineCrawler()
urls_to_crawl = ['https://example.com', 'https://another.example.org']
await crawler.run(urls_to_crawl)
```
在这个例子中,`CoroutineCrawler`是一个协程类,它包含两个异步方法:`fetch_page`用于发起网络请求,`parse_data`负责数据的初步解析。`run`方法将所有URL作为任务并行执行,获取响应后再依次调用`parse_data`。
阅读全文
相关推荐
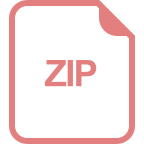
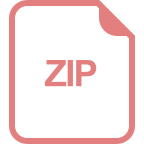
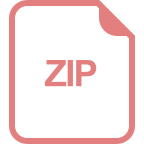


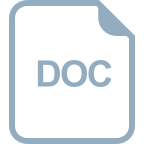
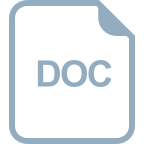

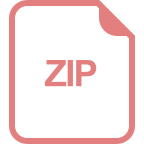
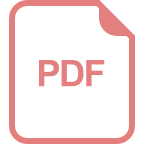
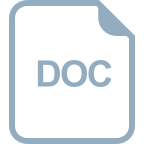
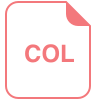
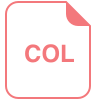
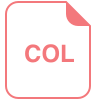

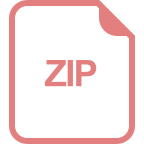
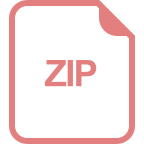
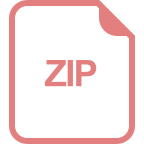