银行业务队列简单模拟c语言
时间: 2024-10-13 22:01:32 浏览: 66
在C语言中,我们可以使用结构体、指针以及队列数据结构来简单模拟银行业务队列。下面是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// 定义银行账户结构体
typedef struct {
int account_number; // 账户号码
float balance; // 当前余额
} BankAccount;
// 定义队列结构体
typedef struct Queue {
BankAccount* items;
int size;
int front;
int rear;
} Queue;
// 初始化队列
void initQueue(Queue* queue) {
queue->items = (BankAccount*)malloc(sizeof(BankAccount));
queue->size = 0;
queue->front = -1;
queue->rear = -1;
}
// 添加元素到队尾
void enqueue(Queue* queue, BankAccount account) {
if (queue->size == queue->rear + 1) { // 如果队满
queue->items = (BankAccount*)realloc(queue->items, (queue->size + 1) * sizeof(BankAccount)); // 扩容
queue->rear++;
} else {
queue->rear++;
}
queue->items[queue->rear] = account;
}
// 从队头移除并返回元素
BankAccount dequeue(Queue* queue) {
if (queue->front == -1 || queue->front >= queue->rear) { // 如果队空
return (BankAccount){0, 0}; // 返回无效操作
}
BankAccount account = queue->items[queue->front];
queue->front++; // 移动队头
if (queue->front == queue->rear) { // 如果队空
free(queue->items);
queue->items = NULL;
} else {
queue->items = (BankAccount*)realloc(queue->items, queue->size * sizeof(BankAccount)); // 缩小队列大小
}
return account;
}
int main() {
Queue bank_queue;
initQueue(&bank_queue);
// 模拟业务添加和处理
BankAccount deposit = {123456, 1000.0};
enqueue(&bank_queue, deposit);
BankAccount withdrawal = dequeue(&bank_queue);
printf("Withdrawn Account Number: %d, Balance: %.2f\n", withdrawal.account_number, withdrawal.balance);
return 0;
}
```
在这个例子中,我们创建了一个银行账户队列,可以进行存款(deposit)和取款(withdrawal)的操作。这只是一个基础的模拟,实际的银行系统会更复杂,包括并发控制、错误处理等。
阅读全文
相关推荐

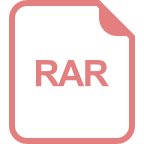
















