onnxruntime gpu c++
时间: 2023-09-06 09:05:04 浏览: 230
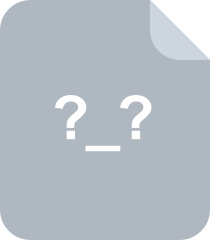
C++使用onnxruntime/opencv对onnx模型进行推理(附代码)

ONNX Runtime是一个用于高性能推理的机器学习推理引擎,支持在GPU上加速推理任务。通过使用ONNX Runtime GPU C API,开发者可以直接在C程序中使用ONNX Runtime进行在GPU上进行推理。
ONNX Runtime GPU C API提供了一系列函数和数据结构,开发者可以用它来加载已经训练好的模型,并将输入数据传入模型进行推理。在GPU上进行推理可以显著提高推理的速度和效率,特别是对于模型和数据较大的情况。
为了使用ONNX Runtime GPU C API,开发者需要将其包含在自己的C程序中,并按照指定的接口进行调用。开发者需要连接到GPU并创建一个ONNX Runtime的推理环境,然后加载模型,并为输入数据分配内存并传入模型进行推理。最后,开发者可以获取输出的预测结果。
通过使用ONNX Runtime GPU C API,开发者可以有效地利用GPU的并行计算能力来加速推理任务。这对于需要处理大规模数据或复杂模型的机器学习应用来说是非常有益的。同时,ONNX Runtime还支持跨多个GPU的分布式推理,可以进一步提高推理的性能和吞吐量。
总之,ONNX Runtime GPU C API提供了一个高性能的机器学习推理引擎,支持在GPU上加速推理任务。开发者可以使用它来加速推理过程,提高效率,并应用在各种机器学习应用中。
阅读全文
相关推荐
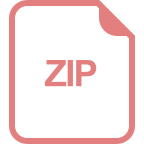
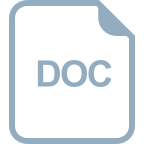
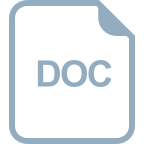
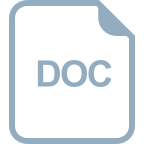




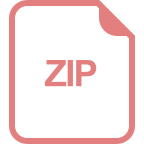
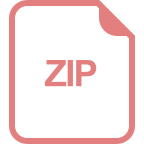
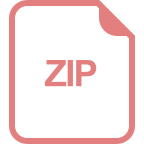
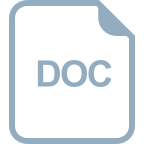
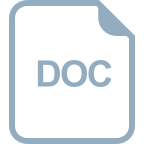
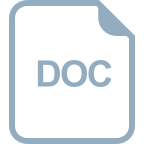
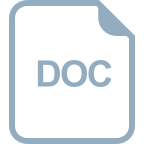

