现有一个整型数组{10,20,30,40,50,60,70,80,90,100},使用随机文件流将其存入文件number.txt中,然后将数据读取出来并输出。
时间: 2024-10-20 20:08:09 浏览: 15
在Python中,你可以使用内置的`random`模块生成随机文件名,并结合`pickle`模块来处理序列数据,如整数数组。以下是步骤:
1. **向文件写入**:
```python
import random
import pickle
# 整型数组
numbers = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
filename = 'number.txt' + str(random.randint(1, 1000)) # 随机文件名避免覆盖原有文件
# 使用pickle将数据序列化并写入文件
with open(filename, 'wb') as f:
pickle.dump(numbers, f)
```
2. **从文件读取并输出**:
```python
# 从文件中读取数据
with open(filename, 'rb') as f:
loaded_numbers = pickle.load(f)
# 输出读取的数据
for number in loaded_numbers:
print(number)
```
这里我们假设`number.txt`目录下可以写入文件。如果不希望每次都生成新的文件名,可以直接使用固定文件名,只是在运行程序时可能会有冲突的风险。
相关问题
JAVA现有一个整型数组{10,20,30,40,50,60,70,80,90,100},使用随机文件流将其存入文件number.txt中,然后将数据读取出来并输出。
在Java中,你可以使用`java.io`包下的`FileWriter`、`BufferedWriter`和`RandomAccessFile`来实现这个功能。以下是步骤:
1. **将整型数组写入文件**:
```java
import java.io.*;
import java.util.Arrays;
public class ArrayToFile {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50, 60, 70, 80, 90, 100};
String fileName = "number.txt";
try (FileWriter writer = new FileWriter(fileName);
BufferedWriter bufferWriter = new BufferedWriter(writer)) {
// 将数组转换为字符串,逗号分隔
for (int number : numbers) {
bufferWriter.write(number + ",");
}
// 删除最后一个逗号
bufferWriter.write("\n");
bufferWriter.flush();
} catch (IOException e) {
System.err.println("Error writing to file: " + e.getMessage());
}
}
}
```
2. **从文件中读取数据并输出**:
```java
public class ReadFile {
public static void main(String[] args) {
String fileName = "number.txt";
try (RandomAccessFile reader = new RandomAccessFile(fileName, "r")) {
long length = reader.length(); // 获取文件长度
byte[] bytes = new byte[(int) length];
reader.readFully(bytes);
// 使用字符串处理工具解析二进制数据
String readString = new String(bytes, StandardCharsets.UTF_8);
String[] numbersStr = readString.split(",");
for (String numberStr : numbersStr) {
System.out.println(Integer.parseInt(numberStr));
}
} catch (IOException e) {
System.err.println("Error reading from file: " + e.getMessage());
}
}
}
```
运行这两个程序,首先会把数组存储到文件中,然后读取文件内容并打印。
现有一个文件input.txt,内容如下: N=x(x为从2到100的随机数字) 1 2 2 1 2 7 4 5 3……(一共有2到100个随机数字,数字大小在0到9之间) 请编写一段c++代码,定义一个int型变量n,将第一行“N=”后面的数字赋值给n,定义一个int型变量length,统计第二行中所有数字的个数,将其值赋给length,定义一个整型数组a[length],将第二行中的所有数字依次赋值给a[i](i=0,1,2…length-1)如果文件中第二行出现了字母、负数、大于10的数、汉字,指出错误并终止程序(报错信息使用汉字)
可以使用以下的C++代码实现你的要求:
```cpp
#include <iostream>
#include <fstream>
#include <cstdlib>
using namespace std;
int main() {
ifstream inputFile("input.txt");
if (!inputFile) {
cout << "无法打开文件!" << endl;
return 1;
}
string line1, line2;
getline(inputFile, line1);
getline(inputFile, line2);
inputFile.close();
// 获取N的值
int n;
size_t pos = line1.find("N=");
if (pos != string::npos) {
n = atoi(line1.substr(pos + 2).c_str());
} else {
cout << "文件内容格式错误!" << endl;
return 1;
}
// 统计数字个数
int length = 0;
for (char c : line2) {
if (isdigit(c)) {
length++;
} else if (c != ' ') {
cout << "文件内容格式错误!" << endl;
return 1;
}
}
// 检查数字范围并赋值给数组
int* a = new int[length];
int i = 0;
pos = 0;
while (i < length) {
int digit = atoi(line2.substr(pos).c_str());
if (digit < 0 || digit > 9) {
cout << "文件内容格式错误!" << endl;
delete[] a;
return 1;
}
a[i] = digit;
pos = line2.find(' ', pos) + 1;
if (pos == string::npos) {
break;
}
i++;
}
// 输出结果
cout << "N的值为:" << n << endl;
cout << "数字个数为:" << length << endl;
cout << "数组内容为:";
for (i = 0; i < length; i++) {
cout << a[i] << " ";
}
cout << endl;
delete[] a;
return 0;
}
```
在代码中,我们首先打开文件并逐行读取内容。然后,我们根据第一行中的"N="获取N的值,并使用`atoi`函数将其转换为整数。接下来,我们统计第二行中的数字个数,并检查每个数字是否在0到9之间。最后,我们将第二行中的数字依次赋值给数组a,并输出结果。
请确保将代码保存为`.cpp`文件,并在同一目录下创建一个名为`input.txt`的文本文件,将问题描述中的内容复制到该文件中。运行代码后,你将得到N的值、数字个数以及数组内容的输出结果。如果文件格式不符合要求,程序将报错并终止。
希望能对你有所帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐
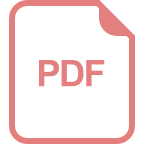
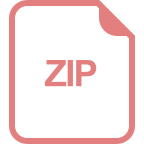
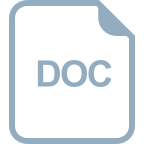
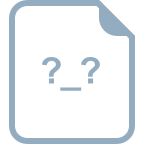
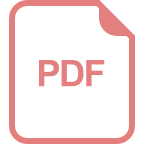
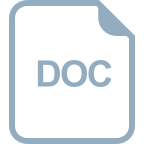
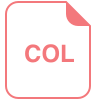
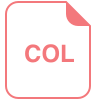
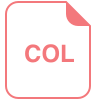
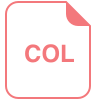
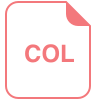
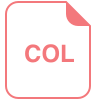
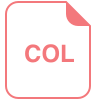
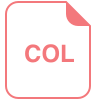
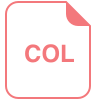
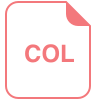
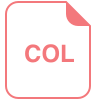