pandas在groupby后会有level_0
时间: 2023-09-10 18:02:55 浏览: 64
在使用pandas进行groupby操作后,会出现level_0这个列名。这是因为在groupby操作中,如果groupby的列是多层次的索引(MultiIndex),则会生成一个新的DataFrame,将groupby的索引作为新生成DataFrame的列,名为level_0。level_0列是一个整数列,表示的是原始数据中的层次化索引中的第一级索引的值。
例如,如果我们有一个DataFrame包含学生的姓名、科目和成绩,然后我们将它groupby根据科目进行分组,结果将会是一个具有MultiIndex的DataFrame,其中level_0列将会是原始数据中科目的索引值。
这个level_0列可以用于后续的数据分析和操作,比如筛选特定的组,计算每个组的统计指标等。如果我们不需要level_0列,可以通过reset_index()方法将其转换为普通的整数索引,使得结果DataFrame没有MultiIndex,而是直接使用默认的整数索引。
相关问题
pandas中groupby
Pandas中的groupby函数可以用于对数据进行分组和聚合操作,常用于数据分析和统计。groupby函数的一般用法为:
```
DataFrame.groupby(by=None, axis=0, level=None, as_index=True, sort=True, group_keys=True, squeeze=False, observed=False, **kwargs)
```
其中,最常用的参数为by,它可以指定按照哪些列进行分组。例如:
```
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie', 'Alice', 'Bob', 'Charlie'],
'Subject': ['Math', 'Math', 'Math', 'Science', 'Science', 'Science'],
'Score': [80, 90, 85, 95, 92, 89]}
df = pd.DataFrame(data)
# 按照Name列进行分组,并计算每个分组的平均值
result = df.groupby('Name').mean()
print(result)
```
输出结果为:
```
Score
Name
Alice 87.5
Bob 91.0
Charlie 87.0
```
上述代码中,我们按照Name列进行分组,并对每个分组的Score列求均值。最终得到了每个人的平均成绩。需要注意的是,groupby函数返回的是一个GroupBy对象,我们可以对其进行各种聚合操作,例如mean、sum、count等。
pandas groupby
Pandas groupby is a powerful function in the Pandas library that allows us to group data based on some criteria and perform various computations on each group. It splits the data into groups based on the selected criteria and then applies the desired function to each group.
The syntax for the groupby function is as follows:
```
df.groupby(by=None, axis=0, level=None, as_index=True, sort=True, group_keys=True, squeeze=False, observed=False, dropna=True)
```
Where:
- by: This parameter specifies the column or list of columns based on which the grouping will be done.
- axis: This parameter specifies the axis along which the grouping will be done. By default, it is 0 (row-wise grouping).
- level: This parameter is used to specify the level (if the data is multi-indexed) on which the grouping will be done.
- as_index: This parameter is used to specify whether to return the grouped by columns as the index of the resulting DataFrame (True by default).
- sort: This parameter is used to specify whether to sort the result by the group keys (True by default).
- group_keys: This parameter is used to specify whether to add group keys to the index to identify the group (True by default).
- squeeze: This parameter is used to specify whether to return a Series if possible (False by default).
- observed: This parameter is used to specify whether to only group by observed values in the data (False by default).
- dropna: This parameter is used to specify whether to exclude missing values from the grouping (True by default).
Here's an example of how to use the groupby function:
```
import pandas as pd
# Creating a DataFrame
data = {'Name': ['John', 'Sam', 'John', 'Marry', 'Sam', 'Marry'],
'Subject': ['Math', 'Science', 'Math', 'Science', 'Math', 'Science'],
'Score': [80, 90, 75, 85, 95, 80]}
df = pd.DataFrame(data)
# Grouping the DataFrame by the 'Name' column and calculating the mean score for each group
grouped_df = df.groupby('Name')['Score'].mean()
print(grouped_df)
```
Output:
```
Name
John 77.5
Marry 82.5
Sam 92.5
Name: Score, dtype: float64
```
In this example, we grouped the DataFrame by the 'Name' column and then calculated the mean score for each group using the mean function. The resulting DataFrame shows the mean score for each group.
相关推荐
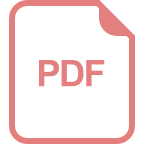
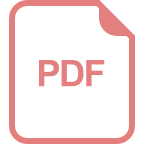
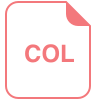











