python实现transformer算法
时间: 2024-04-25 15:19:50 浏览: 211
Transformer算法是一种用于自然语言处理和机器翻译等任务的重要模型。它由Google在2017年提出,并在机器翻译任务中取得了显著的成果。下面是Python实现Transformer算法的一般步骤:
1. 导入必要的库:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
```
2. 定义Transformer模型的基本组件,包括自注意力机制(self-attention)、前馈神经网络(feed-forward network)和残差连接(residual connection):
```python
class SelfAttention(nn.Module):
def __init__(self, d_model, n_heads):
super(SelfAttention, self).__init__()
self.d_model = d_model
self.n_heads = n_heads
self.head_dim = d_model // n_heads
self.query = nn.Linear(d_model, d_model)
self.key = nn.Linear(d_model, d_model)
self.value = nn.Linear(d_model, d_model)
def forward(self, x):
batch_size = x.shape
q = self.query(x)
k = self.key(x)
v = self.value(x)
q = q.view(batch_size, -1, self.n_heads, self.head_dim).transpose(1, 2)
k = k.view(batch_size, -1, self.n_heads, self.head_dim).transpose(1, 2)
v = v.view(batch_size, -1, self.n_heads, self.head_dim).transpose(1, 2)
scores = torch.matmul(q, k.transpose(-2, -1)) / torch.sqrt(torch.tensor(self.head_dim, dtype=torch.float32))
attention_weights = F.softmax(scores, dim=-1)
x = torch.matmul(attention_weights, v)
x = x.transpose(1, 2).contiguous().view(batch_size, -1, self.d_model)
return x
class FeedForward(nn.Module):
def __init__(self, d_model, d_ff):
super(FeedForward, self).__init__()
self.linear1 = nn.Linear(d_model, d_ff)
self.linear2 = nn.Linear(d_ff, d_model)
def forward(self, x):
x = F.relu(self.linear1(x))
x = self.linear2(x)
return x
class ResidualConnection(nn.Module):
def __init__(self, d_model, dropout_rate):
super(ResidualConnection, self).__init__()
self.dropout = nn.Dropout(dropout_rate)
self.layer_norm = nn.LayerNorm(d_model)
def forward(self, x, sublayer):
return x + self.dropout(sublayer(self.layer_norm(x)))
```
3. 定义Transformer模型的编码器和解码器:
```python
class EncoderLayer(nn.Module):
def __init__(self, d_model, n_heads, d_ff, dropout_rate):
super(EncoderLayer, self).__init__()
self.self_attention = SelfAttention(d_model, n_heads)
self.feed_forward = FeedForward(d_model, d_ff)
self.residual_connection = ResidualConnection(d_model, dropout_rate)
def forward(self, x):
x = self.residual_connection(x, lambda x: self.self_attention(x))
x = self.residual_connection(x, lambda x: self.feed_forward(x))
return x
class Encoder(nn.Module):
def __init__(self, d_model, n_heads, d_ff, dropout_rate, n_layers):
super(Encoder, self).__init__()
self.layers = nn.ModuleList([EncoderLayer(d_model, n_heads, d_ff, dropout_rate) for _ in range(n_layers)])
def forward(self, x):
for layer in self.layers:
x = layer(x)
return x
class DecoderLayer(nn.Module):
def __init__(self, d_model, n_heads, d_ff, dropout_rate):
super(DecoderLayer, self).__init__()
self.self_attention = SelfAttention(d_model, n_heads)
self.encoder_attention = SelfAttention(d_model, n_heads)
self.feed_forward = FeedForward(d_model, d_ff)
self.residual_connection = ResidualConnection(d_model, dropout_rate)
def forward(self, x, encoder_output):
x = self.residual_connection(x, lambda x: self.self_attention(x))
x = self.residual_connection(x, lambda x: self.encoder_attention(x))
x = self.residual_connection(x, lambda x: self.feed_forward(x))
return x
class Decoder(nn.Module):
def __init__(self, d_model, n_heads, d_ff, dropout_rate, n_layers):
super(Decoder, self).__init__()
self.layers = nn.ModuleList([DecoderLayer(d_model, n_heads, d_ff, dropout_rate) for _ in range(n_layers)])
def forward(self, x, encoder_output):
for layer in self.layers:
x = layer(x, encoder_output)
return x
```
4. 定义Transformer模型:
```python
class Transformer(nn.Module):
def __init__(self, src_vocab_size, tgt_vocab_size, d_model, n_heads, d_ff, dropout_rate, n_layers):
super(Transformer, self).__init__()
self.encoder = Encoder(d_model, n_heads, d_ff, dropout_rate, n_layers)
self.decoder = Decoder(d_model, n_heads, d_ff, dropout_rate, n_layers)
self.src_embedding = nn.Embedding(src_vocab_size, d_model)
self.tgt_embedding = nn.Embedding(tgt_vocab_size, d_model)
self.fc = nn.Linear(d_model, tgt_vocab_size)
def forward(self, src_input, tgt_input):
src_embedded = self.src_embedding(src_input)
tgt_embedded = self.tgt_embedding(tgt_input)
encoder_output = self.encoder(src_embedded)
decoder_output = self.decoder(tgt_embedded, encoder_output)
output = self.fc(decoder_output)
return output
```
以上是一个简单的Python实现Transformer算法的示例。你可以根据具体任务的需求进行修改和扩展。如果你有任何问题,请随时提问。
阅读全文
相关推荐






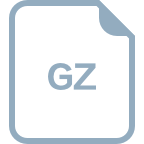









