现在有一个按钮选择文件,如何选择完就在页面输出指定路径下得txt文件,请给出前后端的完整代码
时间: 2024-10-11 21:11:04 浏览: 23
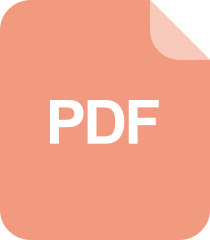
json格式的Ajax提交示例代码
前端通常使用JavaScript配合HTML来创建一个文件选择功能,并通过Ajax将选中的文件路径发送到后台处理。这里是一个简单的示例:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>File Selection</title>
</head>
<body>
<input type="file" id="fileInput" accept=".txt" />
<button onclick="selectAndShow()">选择并显示</button>
<script>
function selectAndShow() {
const input = document.getElementById('fileInput');
if (input.files.length) {
const file = input.files[0];
// 这里只是模拟发送到服务器,实际应用中请替换为AJAX请求
const reader = new FileReader();
reader.onload = function(event) {
const txtContent = event.target.result;
alert("文本内容:\n" + txtContent);
// 输出到页面或其他地方需要额外的DOM操作
// 比如:
// document.getElementById('outputArea').innerText = txtContent;
};
reader.readAsText(file); // 读取文件内容为文本
} else {
alert("请选择文件");
}
}
</script>
</body>
</html>
```
在这个例子中,用户选择了一个`.txt`文件后,点击“选择并显示”按钮,会触发`selectAndShow`函数。这个函数获取输入框中的文件,然后使用`FileReader`读取文件的内容。读取完成后,它会在浏览器的警告框中显示文本内容。
至于后端,假设你使用Node.js和Express框架,接收文件路径后可以这么处理:
```javascript
const express = require('express');
const app = express();
app.post('/upload', (req, res) => {
const filePath = req.body.filePath; // 假设从前端POST过来的路径
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) {
console.error(err);
return res.status(500).send('文件读取错误');
}
res.send(data);
});
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
在上面的后端代码中,你需要安装`fs`模块 (`npm install fs`),`fs.readFile`用于读取服务器上的文件内容。
阅读全文
相关推荐
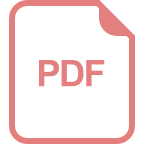
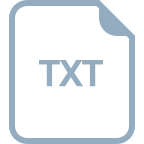
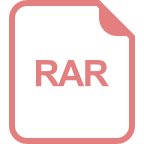
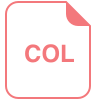
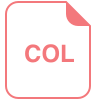
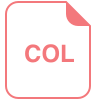
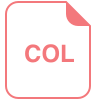
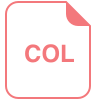
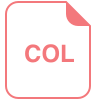
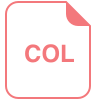
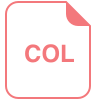
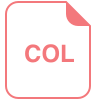
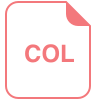
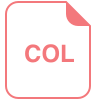
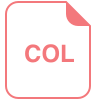
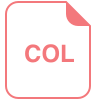

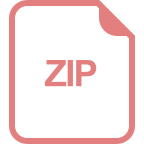