帮我用python 利用摄像头识别手掌关键点的程序
时间: 2024-09-16 10:01:39 浏览: 52
使用Python和OpenCV库来识别摄像头中的手掌关键点,通常涉及到人体姿态估计(Hand Pose Estimation)技术,这里我们可以使用深度学习模型,比如Mediapipe(原Android团队开发)。以下是一个基本的步骤指南:
首先,你需要安装必要的库:
```bash
pip install opencv-python mediapipe numpy Pillow
```
然后,编写Python代码:
```python
import cv2
import mediapipe as mp
# 加载Mediapipe手部追踪模型
mp_hands = mp.solutions.hands
hands = mp_hands.Hands(min_detection_confidence=0.5, min_tracking_confidence=0.5)
# 初始化摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取摄像头帧
success, frame = cap.read()
if not success:
break
# 转换到灰度图像以提高性能
gray_frame = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 进行手势检测
results = hands.process(gray_frame)
# 如果有检测到手
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
# 提取关键点坐标
landmarks = []
for landmark in hand_landmarks.landmark:
x, y = int(landmark.x * frame.shape[1]), int(landmark.y * frame.shape[0])
landmarks.append((x, y))
# 绘制关键点
cv2.draw_landmarks(frame, hand_landmarks, mp_hands.HAND_CONNECTIONS, (255, 0, 0), cv2.LINE_AA)
# 显示结果
cv2.imshow("Hand Landmarks", frame)
# 按 'q' 键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
这个程序会显示实时的摄像头画面,其中标出了每个手掌的关键点。注意,Mediapipe的手部追踪模型需要网络支持,因为它是基于云端预训练模型的。
阅读全文
相关推荐
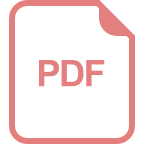
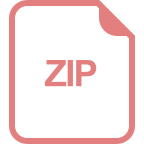
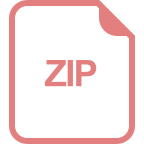


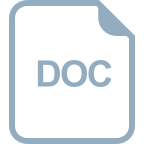


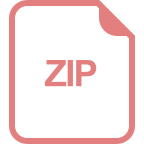
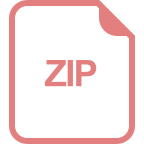
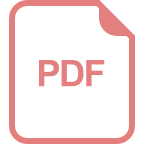
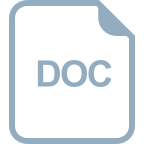
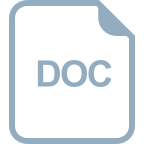
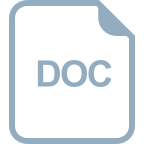
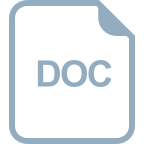
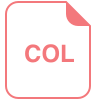



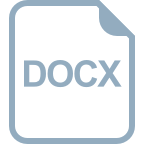