用python语言编写一个摄像头识别手掌关键点的代码,并注明需要哪些包
时间: 2024-09-15 16:11:04 浏览: 42
要在Python中使用摄像头识别手掌的关键点,通常我们会利用OpenCV(cv2库)和MediaPipe(mediapipe库)。以下是一个简单的示例代码:
```python
import cv2
import mediapipe as mp
# 加载预定义的手掌模型
mp_hands = mp.solutions.hands
hands = mp_hands.Hands()
# 设置视频捕获设备(0表示默认摄像头)
cap = cv2.VideoCapture(0)
while True:
# 读取一帧
success, frame = cap.read()
if not success:
break
# 转为灰度图像以便于处理
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 对灰度图像进行手部检测
results = hands.process(gray)
# 如果检测到手,绘制关键点
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
for landmark in hand_landmarks.landmark:
# 获取关键点坐标并标记
x, y = int(landmark.x * frame.shape[1]), int(landmark.y * frame.shape[0])
cv2.circle(frame, (x, y), 5, (255, 0, 0), cv2.FILLED)
# 显示结果
cv2.imshow('Hand Detection', frame)
# 按 'q' 键退出循环
if cv2.waitKey(10) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
这个代码需要的包有:
1. `opencv-python`(OpenCV库,用于视频捕捉和图像处理)
2. `mediapipe`(Google的MediaPipe库,用于手势识别)
阅读全文
相关推荐
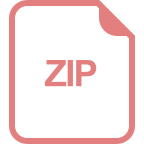
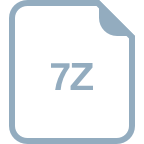
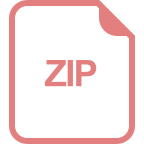

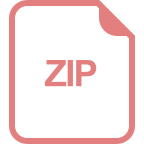
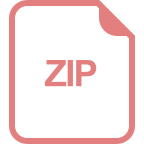
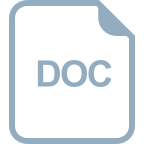
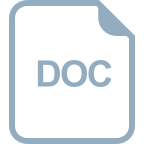
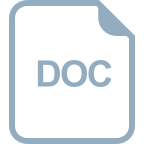
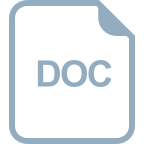
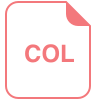
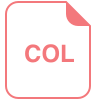

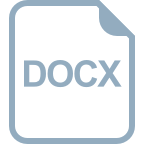
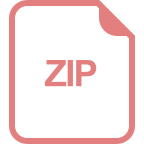