建立一个线性表,元素由使用者输入,能够实现插入,删除,以及输出指定位置的元素的功能
时间: 2024-10-16 19:14:54 浏览: 25
建立一个线性表,通常可以使用数组或链表作为数据结构。这里以链表为例来说明,因为链表可以动态添加和删除元素,而数组则受限于固定大小。
**1. 链表实现:**
链表是由一系列节点组成,每个节点包含一个数据元素和指向下一个节点的指针。首先,你需要创建一个节点类(Node),然后定义一个链表类(List):
```python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class List:
def __init__(self):
self.head = None
# 插入元素
def insert(self, position, data):
if position < 0 or position > len(self):
print("Invalid position")
return
new_node = Node(data)
if position == 0:
new_node.next = self.head
self.head = new_node
else:
current = self.head
for _ in range(position - 1):
current = current.next
new_node.next = current.next
current.next = new_node
# 删除元素
def delete(self, position):
if position < 0 or position >= len(self):
print("Invalid position")
return
if position == 0:
self.head = self.head.next
else:
current = self.head
for _ in range(position - 1):
current = current.next
current.next = current.next.next
# 输出指定位置的元素
def get_element(self, position):
if position < 0 or position >= len(self):
print("Invalid position")
return None
current = self.head
for _ in range(position):
current = current.next
return current.data
# 获取链表长度
def __len__(self):
count = 0
current = self.head
while current:
count += 1
current = current.next
return count
```
阅读全文
相关推荐
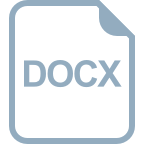
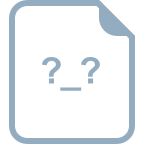
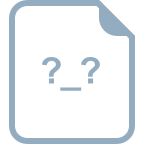

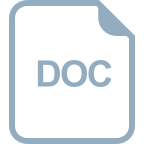
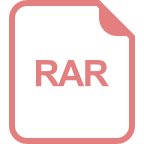
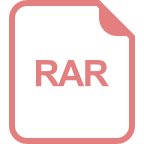
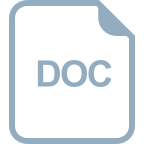
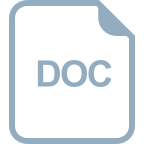
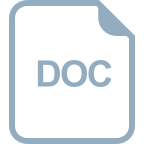
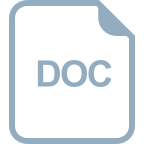
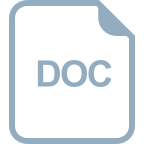
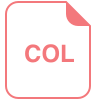
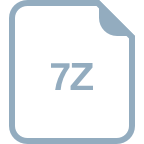
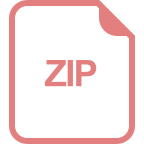
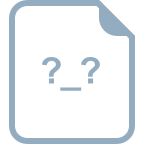
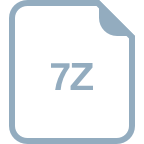
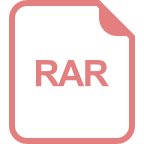
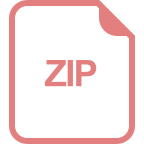