使用python语言构造一个类,实现通过SNMP采集设备的名称、IP地址、配置等信息并存储在mysql数据库中
时间: 2024-04-16 10:27:20 浏览: 24
当然可以!以下是一个示例代码,展示了何使用Python语言构建一个类来实现通过SNMP采集设备信息并存储到MySQL数据库中:
```python
import mysql.connector
from pysnmp.hlapi import *
class SNMPDeviceCollector:
def __init__(self, host, community):
self.host = host
self.community = community
self.db = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
def collect_device_info(self):
# 创建SNMP请求
g = getCmd(
SnmpEngine(),
CommunityData(self.community),
UdpTransportTarget((self.host, 161)),
ContextData(),
ObjectType(ObjectIdentity('SNMPv2-MIB', 'sysName', 0)),
ObjectType(ObjectIdentity('SNMPv2-MIB', 'ipAdEntAddr'))
)
# 发送SNMP请求并处理响应
error_indication, error_status, error_index, var_binds = next(g)
if error_indication:
print(f"SNMP error: {error_indication}")
return
if error_status:
print(f"SNMP error: {error_status.prettyPrint()} at {error_index and var_binds[int(error_index)-1][0] or '?'}")
return
# 解析SNMP响应并存储到数据库
sys_name = var_binds[0][1]
ip_address = var_binds[1][1]
config = self.get_device_config()
cursor = self.db.cursor()
sql = "INSERT INTO devices (name, ip_address, config) VALUES (%s, %s, %s)"
val = (sys_name, ip_address, config)
cursor.execute(sql, val)
self.db.commit()
def get_device_config(self):
# 在此处实现获取设备配置的逻辑
# 可以使用pysnmp或其他工具来获取配置信息
# 返回设备配置字符串
pass
# 使用示例
collector = SNMPDeviceCollector("192.168.1.1", "public")
collector.collect_device_info()
```
请注意,上述代码只是一个示例,需要根据实际情况进行适当修改。你需要安装并导入 `mysql.connector` 和 `pysnmp` 库,以便在Python中连接MySQL数据库和执行SNMP操作。
此示例代码中的 `collect_device_info` 方法使用SNMP协议从设备中获取名称和IP地址,并调用 `get_device_config` 方法来获取设备的配置信息(请根据实际情况自行实现该方法)。然后,它将这些信息存储到MySQL数据库中。
记得在代码中替换 `yourusername`、`yourpassword` 和 `yourdatabase` 为你的MySQL数据库的实际凭据和数据库名称。
希望这个示例对你有所帮助!如有任何问题,请随时提问。
相关推荐
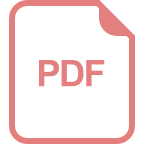
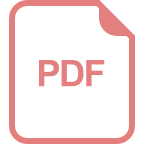
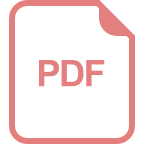














